Service(服務)在Android中地位是至關重要的,我們可以通過Activity與Broadcast(廣播)啟動Service(服務),我們本章學習如何通過廣播Broadcast啟動服務Service。
也許你會說,能用Activity啟動,干嘛要用廣播呢?——且聽電話監聽、短信監聽再作分解!
一、設計界面
1、布局文件
打開res/layout/activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
- <Button
- android:id="@+id/send"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="通過Broadcast啟動Service" />
-
- </LinearLayout>
二、程序文件
1、創建“src/com.genwoxue.broadcastservice/ServiceUtil.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.broadcastservice;
-
- import android.app.Service;
- import android.content.Intent;
- import android.os.IBinder;
- import android.util.Log;
-
- public class ServiceUtil extends Service{
-
- private static final String TAG="AboutService";
- @Override
- public IBinder onBind(Intent intent){
- return null;
- }
-
- @Override
- public void onCreate(){
- Log.i(TAG,"服務:onCreate()");
- }
-
- //啟動
- @Override
- public int onStartCommand(Intent intent,int flags,int startId){
- Log.i(TAG, "服務啟動:onStart()=>Intent"+intent+",startID="+startId);
- return Service.START_CONTINUATION_MASK;
- }
-
- @Override
- public void onDestroy(){
- Log.i(TAG,"服務:onDestroy()");
- }
-
- }
2、創建“src/com.genwoxue.broadcastservice/BroadcastReceiverUtil.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.broadcastservice;
-
- import android.content.BroadcastReceiver;
- import android.content.Context;
- import android.content.Intent;
-
- public class BroadcastReceiverUtil extends BroadcastReceiver{
- @Override
- public void onReceive(Context context,Intent intent){
- //廣播接收器(接收方)判斷Action為“com.genwoxue.action.ABOUTSERVICE”則啟動服務
- if("com.genwoxue.action.ABOUTSERVICE".equals(intent.getAction())){
- context.startService(new Intent(context,ServiceUtil.class));
- }
-
- }
- }
3、打開“src/com.genwoxue.broadcastservice/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.broadcastservice;
-
-
- import android.os.Bundle;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.app.Activity;
- import android.content.Intent;
- import android.content.IntentFilter;
-
- public class MainActivity extends Activity {
-
- private BroadcastReceiverUtil util=null;
- private Button btnSend=null;
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- btnSend=(Button)super.findViewById(R.id.send);
- btnSend.setOnClickListener(new OnClickListener(){
- @Override
- public void onClick(View v){
- //發送廣播:其Action為“com.genwoxue.action.ABOUTSERVICE”
- Intent intent=new Intent("com.genwoxue.action.ABOUTSERVICE");
- MainActivity.this.sendBroadcast(intent);
-
-
- //實例化廣播過濾器(只過濾其Action為"com.genwoxue.action.ABOUTSERVICE")
- IntentFilter filter=new IntentFilter("com.genwoxue.action.ABOUTSERVICE");
- //實例化廣播接收器(接收方)
- util=new BroadcastReceiverUtil();
- //注冊BroadcastReceiver:參數為接收器與過濾器
- MainActivity.this.registerReceiver(util, filter);
- }
- });
- }
-
- @Override
- protected void onStop(){
- //停止廣播
- super.unregisterReceiver(util);
- super.onStop();
-
- //停止服務
- Intent intent=new Intent(MainActivity.this,ServiceUtil.class);
- MainActivity.this.stopService(intent);
- }
-
- }
三、配置文件
打開“AndroidManifest.xml”文件。
然後輸入以下代碼:
[java] view plain copy
- <?xml version="1.0" encoding="utf-8"?>
- <manifest xmlns:android="http://schemas.android.com/apk/res/android"
- package="com.genwoxue.broadcastservice"
- android:versionCode="1"
- android:versionName="1.0" >
-
- <uses-sdk
- android:minSdkVersion="10"
- android:targetSdkVersion="15" />
-
- <application
- android:allowBackup="true"
- android:icon="@drawable/ic_launcher"
- android:label="@string/app_name"
- android:theme="@style/AppTheme" >
- <activity
- android:name="com.genwoxue.broadcastservice.MainActivity"
- android:label="@string/app_name" >
- <intent-filter>
- <action android:name="android.intent.action.MAIN" />
- <category android:name="android.intent.category.LAUNCHER" />
- </intent-filter>
- </activity>
- <service android:name="com.genwoxue.broadcastservice.ServiceUtil" />
- </application>
-
- </manifest>
注意:需要在AndroidManifest.xml文件中添加<service...>:
<service android:name="com.genwoxue.broadcastservice.ServiceUtil" />
四、運行結果
Activity發送廣播—>廣播接收方啟動Service服務
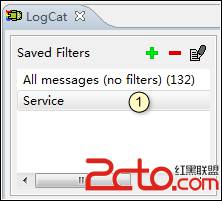
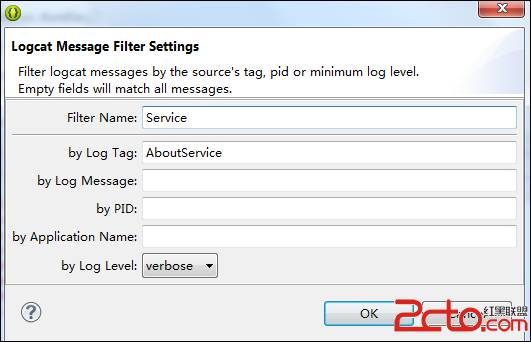
