JSON定義:(javascript object Notation的簡稱)一種輕量級的數據交換格式,具有良好的可讀和便於快速編寫的特性,可以在不同平台間進行數據交換。
1、JSON特點:
(1)json數據是一系列鍵值對的集合;
(2)json已經被大多數開發人員,在網絡數據的傳輸當中應用非常廣泛;
(3)json相對於xml來講解析稍微方便一些。
2、JSON與XML比較
(1)json和xml的數據可讀性基本相同;
(2)json和xml同樣擁有豐富的解析手段;
(3)json相對於xml來講,數據體積小;
(4)json與javascrpit的交互更加方便;
(5)json對數據的描述性相對較差;
(6)JSON的速度要遠遠快於XML。
一、設計界面
1、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:orientation="vertical" >
-
- <Button
- android:id="@+id/save"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="保存JSON文件" />
-
- <Button
- android:id="@+id/read"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="讀取JSON文件" />
-
- </LinearLayout>
二、程序文件
打開“src/com.genwoxue.filejson/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.filejson;
-
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileNotFoundException;
- import java.io.FileOutputStream;
- import java.io.InputStream;
- import java.io.PrintStream;
- import java.util.ArrayList;
- import java.util.HashMap;
- import java.util.Iterator;
- import java.util.List;
- import java.util.Map;
- import org.json.JSONArray;
- import org.json.JSONException;
- import org.json.JSONObject;
- import android.os.Bundle;
- import android.os.Environment;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.Toast;
- import android.app.Activity;
-
- public class MainActivity extends Activity {
-
-
- private Button btnSave=null;
- private Button btnRead=null;
- private File file=null;
- private static final String FILENAME="json.txt";
- private StringBuffer info=null;
-
- private String bookName[]=new String[]{"跟我學Android","從零開始學Android"};
- private String author[]=new String[]{"蔣老夫子","蔣老夫子"};
- private String publisher[]=new String[]{"人民郵電出版社","清華大學出版社"};
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
-
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- btnSave=(Button)super.findViewById(R.id.save);
- btnRead=(Button)super.findViewById(R.id.read);
-
- btnSave.setOnClickListener(new OnClickListener(){
- public void onClick(View v)
- {
- PrintStream ps=null;
-
- JSONArray bookArray=new JSONArray();
-
- for(int i=0;i<MainActivity.this.bookName.length;i++){
- JSONObject object=new JSONObject();
- try {
- object.put("bookname", MainActivity.this.bookName[i]);
- object.put("author",MainActivity.this.author[i]);
- object.put("publisher",MainActivity.this.publisher[i]);
- } catch (JSONException e) {
- e.printStackTrace();
- }
- bookArray.put(object);
- }
-
- //判斷外部存儲卡是否存在
- if(!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)){
- Toast.makeText(getApplicationContext(), "讀取失敗,SD存儲卡不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //初始化File
- String path=Environment.getExternalStorageDirectory().toString()
- +File.separator
- +"genwoxue"
- +File.separator
- +FILENAME;
- file=new File(path);
-
- //如果當前文件的父文件夾不存在,則創建genwoxue文件夾
- if(!file.getParentFile().exists())
- file.getParentFile().mkdirs();
-
- try {
- ps=new PrintStream(new FileOutputStream(file));
- ps.print(bookArray.toString());
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- } finally{
- if(ps!=null)
- ps.close();
- }
-
- }
- });
-
-
- btnRead.setOnClickListener(new OnClickListener(){
- public void onClick(View v)
- {
- info=new StringBuffer();
-
- //判斷外部存儲卡是否存在
- if(!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)){
- Toast.makeText(getApplicationContext(), "讀取失敗,SD存儲卡不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //判斷文件是否存在
- String path=Environment.getExternalStorageDirectory().toString()+File.separator+"genwoxue"+File.separator+FILENAME;
- file=new File(path);
-
-
- if(!file.exists()){
- Toast.makeText(getApplicationContext(), "XML文件不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //解析JSON文件
- try {
- InputStream input=new FileInputStream(file);
-
- //InputStream轉換為字符串
- StringBuffer buffer = new StringBuffer();
- byte[] b = new byte[4096];
- int n;
- while ((n = input.read(b))!= -1){
- buffer.append(new String(b,0,n));
- }
-
- //JSON解析
- List<Map<String,Object>> books=MainActivity.this.parseJson(buffer.toString());
- Iterator<Map<String,Object>> iter=books.iterator();
-
- //遍歷列表,獲取書籍信息
- while(iter.hasNext()){
- Map<String,Object> map=iter.next();
- info.append(map.get("bookname")).append("☆☆☆\n");
- info.append(map.get("author")).append("☆☆☆\n");
- info.append(map.get("publisher")).append("☆☆☆\n");
- }
- }catch(Exception e){
- e.printStackTrace();
- }
-
- Toast.makeText(getApplicationContext(), info.toString(), Toast.LENGTH_LONG).show();
-
- }
- });
-
- }
-
-
- /*
- * 定義JSON解析方法parseJson()
- */
- public List<Map<String,Object>> parseJson(String s) throws Exception{
- List<Map<String,Object>> list=new ArrayList<Map<String,Object>>();
- JSONArray array=new JSONArray(s);
- for(int i=0;i<array.length();i++){
- Map<String,Object> map=new HashMap<String,Object>();
- JSONObject object=array.getJSONObject(i);
- map.put("bookname",object.getString("bookname"));
- map.put("author", object.getString("author"));
- map.put("publisher", object.getString("publisher"));
- list.add(map);
- }
- return list;
- }
- }
三、配置文件
打開“AndroidManifest.xml”文件。
然後輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8"?>
- <manifest xmlns:android="http://schemas.android.com/apk/res/android"
- package="com.genwoxue.filejson"
- android:versionCode="1"
- android:versionName="1.0" >
-
- <uses-sdk
- android:minSdkVersion="8"
- android:targetSdkVersion="15" />
- <span style="color:#ff0000;"><strong><uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
- <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/></strong>
- </span> <application
- android:allowBackup="true"
- android:icon="@drawable/ic_launcher"
- android:label="@string/app_name"
- android:theme="@style/AppTheme" >
- <activity
- android:name="com.genwoxue.filejson.MainActivity"
- android:label="@string/app_name" >
- <intent-filter>
- <action android:name="android.intent.action.MAIN" />
- <category android:name="android.intent.category.LAUNCHER" />
- </intent-filter>
- </activity>
- </application>
-
- </manifest>
注意:由於要進行讀寫外部存儲卡操作,故而需要在AndroidManifest.xml文件中添加兩項權限:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
四、運行結果
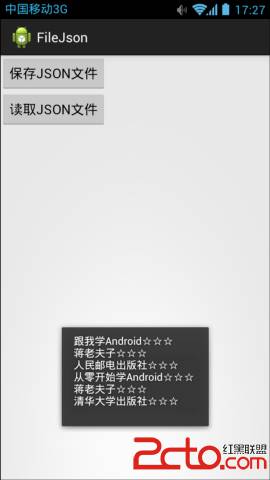