Android有兩種拍照方法,一種是直接調用系統的照相Intent,使用 onActivityResult獲取圖片資源或者指定圖片路徑,拍照返回成功後去指定路徑讀取圖片;一種是用SurfaceView自定義界面,添加業務個性化功能。
一、第一種方法
1、設計界面
(1)、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
- <Button
- android:id="@+id/bysystem"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="調用系統相機不返回結果" />
-
- <Button
- android:id="@+id/byself"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="調用系統相機並返回結果" />
-
- <ImageView
- android:id="@+id/photo"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content" />
-
- </LinearLayout>
2、程序文件
打開“src/com.genwoxue.camera/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.camera;
-
-
- import java.io.File;
- import android.app.Activity;
- import android.content.Intent;
- import android.net.Uri;
- import android.os.Bundle;
- import android.os.Environment;
- import android.provider.MediaStore;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.Toast;
-
- public class MainActivity extends Activity {
-
- private Button btnSystem=null;
- private Button btnSelf=null;
- private File file=null;
- private static final String FILENAME="photo.jpg";
-
- private static String path="";
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- btnSystem=(Button)super.findViewById(R.id.bysystem);
- btnSelf=(Button)super.findViewById(R.id.byself);
-
- //調用系統照相機,不返回結果
- btnSystem.setOnClickListener(new OnClickListener(){
- public void onClick(View v)
- {
- Intent intent = new Intent();
- intent.setAction("android.media.action.STILL_IMAGE_CAMERA");
- startActivity(intent);
- }
- });
-
- //調用系統照相機,返回結果
- btnSelf.setOnClickListener(new OnClickListener(){
- public void onClick(View v)
- {
- //判斷外部存儲卡是否存在
- if(!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)){
- Toast.makeText(getApplicationContext(), "讀取失敗,SD存儲卡不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //判斷文件是否存在
- path=Environment.getExternalStorageDirectory().toString()+File.separator+"genwoxue"+File.separator+FILENAME;
- file=new File(path);
- if(!file.exists()){
- File vDirPath = file.getParentFile();
- vDirPath.mkdirs();
- Toast.makeText(getApplicationContext(), "photo.jpg文件不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- Uri uri = Uri.fromFile(file);
- Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
- intent.putExtra(MediaStore.EXTRA_OUTPUT, uri);
- startActivityForResult(intent, 1);
-
- }
- });
-
- }
-
- }
3、運行結果
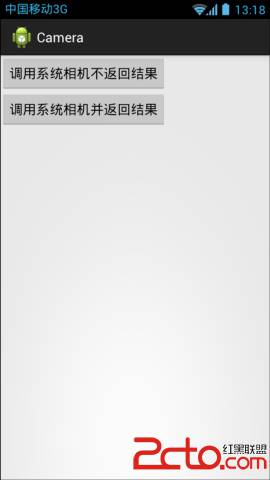
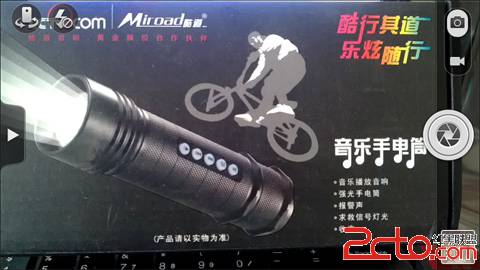
二、第二種方法。
1、設計界面
(1)、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
-
- <Button
- android:id="@+id/byself"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="拍照(自定義相機)" />
-
- <SurfaceView
- android:id="@+id/photo"
- android:layout_width="300dip"
- android:layout_height="400dip" />
-
- </LinearLayout>
2、程序文件
打開“src/com.genwoxue.cameradiy/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.cameradiy;
-
-
- import java.io.BufferedOutputStream;
- import java.io.File;
- import java.io.FileNotFoundException;
- import java.io.FileOutputStream;
- import java.io.IOException;
- import android.app.Activity;
- import android.graphics.Bitmap;
- import android.graphics.BitmapFactory;
- import android.graphics.PixelFormat;
- import android.hardware.Camera;
- import android.hardware.Camera.AutoFocusCallback;
- import android.hardware.Camera.Parameters;
- import android.hardware.Camera.PictureCallback;
- import android.hardware.Camera.ShutterCallback;
- import android.os.Bundle;
- import android.os.Environment;
- import android.util.Log;
- import android.view.SurfaceHolder;
- import android.view.SurfaceView;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.Toast;
-
- public class MainActivity extends Activity {
-
- private Button btnSelf=null;
- private Camera camera=null;
- private static final String TAG="PhotoDIY";
- private String path="";
- private boolean previewRuning=true;
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- //初始化SurfaceView
- SurfaceView mpreview = (SurfaceView) this.findViewById(R.id.photo);
- SurfaceHolder mSurfaceHolder = mpreview.getHolder();
- mSurfaceHolder.addCallback(new SurfaceViewCallback());
- mSurfaceHolder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS);
-
-
- btnSelf=(Button)super.findViewById(R.id.byself);
-
- //拍照(自定義相機)
- btnSelf.setOnClickListener(new OnClickListener(){
- public void onClick(View v)
- {
- if(camera!=null){
- camera.autoFocus(new AutoFocusCallbackimpl());
- }
- }
- });
-
- }
-
- public class SurfaceViewCallback implements SurfaceHolder.Callback{
-
- @Override
- public void surfaceChanged(SurfaceHolder holder,int format,int width,int heith){
-
- }
-
- @Override
- public void surfaceCreated(SurfaceHolder holder){
- //現在智能機可能會有多個鏡頭:一般前置為1;後置為0
- MainActivity.this.camera=Camera.open(0);
- //設置參數
- Parameters param=camera.getParameters();
- param.setPictureFormat(PixelFormat.JPEG);
- param.set("jpeg-quality",85);
- param.setPreviewFrameRate(5);
- camera.setParameters(param);
-
- try {
- camera.setPreviewDisplay(holder); //成像在SurfaceView
- } catch (IOException e) {
- e.printStackTrace();
- }
-
- //開始預覽
- camera.startPreview();
- previewRuning=true;
- }
-
- @Override
- public void surfaceDestroyed(SurfaceHolder holder){
- if(camera!=null){
- if(previewRuning){
- camera.stopPreview();
- previewRuning=false;
- }
- camera.release();
- }
- }
- }
-
- //調用takePicture()方法時,自動執行pictureCallback回調方法
- public PictureCallback picture=new PictureCallback(){
- @Override
- public void onPictureTaken(byte[] data,Camera camera){
- Bitmap bmp=BitmapFactory.decodeByteArray(data, 0, data.length);
- //判斷外部存儲卡是否存在
- if(!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)){
- Toast.makeText(getApplicationContext(), "讀取失敗,SD存儲卡不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //判斷文件是否存在
- path=Environment.getExternalStorageDirectory().toString()
- +File.separator
- +"genwoxue"
- +File.separator
- +System.currentTimeMillis()
- +".jpg";
-
- File file=new File(path);
- if(!file.exists()){
- File vDirPath = file.getParentFile();
- vDirPath.mkdirs();
- Toast.makeText(getApplicationContext(), "photo.jpg文件不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- try {
- BufferedOutputStream bos=new BufferedOutputStream(new FileOutputStream(file));
- bmp.compress(Bitmap.CompressFormat.JPEG, 80, bos);
- try {
- bos.flush();
- bos.close();
- } catch (IOException e) {
- e.printStackTrace();
- }
-
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- }
-
- camera.stopPreview();
- camera.startPreview();
-
- }
- };
-
- //對焦回回調
- public class AutoFocusCallbackimpl implements AutoFocusCallback{
- public void onAutoFocus(boolean success,Camera camera){
-
- if(success){
- camera.takePicture(shutter, null, picture);
- camera.stopPreview();
- }
- }
- }
-
- //快門回調
- public ShutterCallback shutter=new ShutterCallback(){
- public void onShutter(){
-
- }
- };
- }
3、運行結果
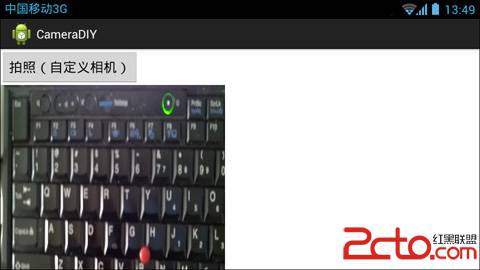