我們可以使用MediaRecorder輕松完成音頻錄音,注意模擬器不支持,需要真機測試。具體步驟如下:
(1)創建一個android.media.MediaRecorder的新實例.
(2)使用MediaRecorder.setAudioSource()設置音頻源,一般要使用MediaRecorder.AudioSource.MIC.
(3)使用MediaRecorder.setOutputFormat()設置輸出文件的格式.
(4)使用MediaRecorder.setOutputFile()設置輸出文件的名字.
(5)使用MediaRecorder.setAudioEncoder()設置音頻編碼.
(6)調用MediaRecorder 實例的MediaRecorder.prepare().
(7)MediaRecorder.start()開始獲取音頻.
(8)調用MediaRecorder.stop().停止.
(9)調用MediaRecorder.release(),就會立即釋放資源.
一、設計界面
1、首先把record.png、stop.png兩張圖片復制到res/drawable-hdpi文件夾內。
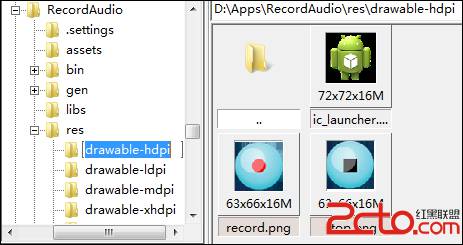
2、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
-
- <ImageButton
- android:id="@+id/record"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/record" />
-
- <ImageButton
- android:id="@+id/stop"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/stop" />
-
-
-
- </LinearLayout>
二、程序文件
打開“src/com.genwoxue.recordaudio/MainActivity.java”文件。
然後輸入以下代碼:
[java] view plain copy
- package com.genwoxue.recordaudio;
-
- import java.io.File;
- import java.io.IOException;
-
- import android.media.MediaRecorder;
- import android.os.Bundle;
- import android.os.Environment;
- import android.util.Log;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.ImageButton;
- import android.widget.Toast;
- import android.app.Activity;
-
- public class MainActivity extends Activity {
-
- private ImageButton btnRecord=null;
- private ImageButton btnStop=null;
-
- private MediaRecorder mRecorder=null;
- private static final String TAG="RECORD";
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- btnRecord=(ImageButton)super.findViewById(R.id.record);
- btnStop=(ImageButton)super.findViewById(R.id.stop);
-
- //開始錄音
- btnRecord.setOnClickListener(new OnClickListener(){
- public void onClick(View v){
- //判斷外部存儲卡是否存在
- if(!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)){
- Toast.makeText(getApplicationContext(), "讀取失敗,SD存儲卡不存在!", Toast.LENGTH_LONG).show();
- return;
- }
-
- //判斷文件是否存在
- String path=Environment.getExternalStorageDirectory().toString()
- +File.separator
- +"genwoxue"
- +File.separator
- +System.currentTimeMillis()
- +".3gp";
-
- mRecorder = new MediaRecorder(); //設置音源為Micphone
- mRecorder.setAudioSource(MediaRecorder.AudioSource.MIC); //設置封裝格式
- mRecorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);
- mRecorder.setOutputFile(path);
- mRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB); //設置編碼格式
-
- try {
- mRecorder.prepare();
- } catch (IOException e) {
- Log.e(TAG, "prepare() failed");
- }
-
- mRecorder.start();
-
- }
-
- });
-
- //停止錄音
- btnStop.setOnClickListener(new OnClickListener(){
- public void onClick(View v){
- mRecorder.stop();
- mRecorder.release();
- mRecorder = null;
- }
- });
- }
-
-
- }
三、運行結果
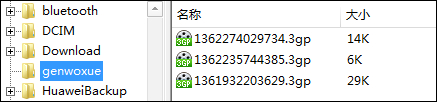
單擊“錄音”按鈕,如右圖所示,將會錄下來,被保存成3gp格式的錄音文件。