在Android中,我們有三種方式來實現視頻的播放:
1、使用其自帶的播放器。指定Action為ACTION_VIEW,Data為Uri,Type為其MIME類型。
2、使用VideoView來播放。在布局文件中使用VideoView結合MediaController來實現對其控制。
3、使用MediaPlayer類和SurfaceView來實現,這種方式很靈活。
本案例著重講解第三種MediaPlayer方式:MediaPlayer播放視頻除了利用MediaPlayer,還需要SurfaceView與之配合,Surface可以完成對後台線程的控制,對於視頻、3D加速或者高頻率對象都有很大的用途。
一、設計界面
1、首先把play.png、stop.png兩張圖片復制到res/drawable-hdpi文件夾內。
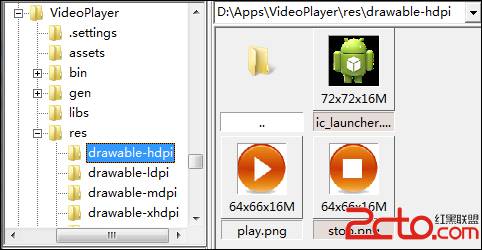
2、布局文件
打開activity_main.xml文件。
輸入以下代碼:
[html] view plain copy
- <?xml version="1.0" encoding="utf-8" ?>
-
- <LinearLayout
- xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
-
- <ImageButton
- android:id="@+id/playvideo"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/play" />
-
- <ImageButton
- android:id="@+id/stopvideo"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/stop" />
-
- <SurfaceView
- android:id="@+id/surface"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content" />
-
- </LinearLayout>
二、程序文件
打開“src/com.genwoxue.mp3/MainActivity.java”文件。
然後輸入以下代碼
[java] view plain copy
- package com.genwoxue.videoplayer;
-
- import java.io.IOException;
-
- import android.app.Activity;
- import android.media.AudioManager;
- import android.media.MediaPlayer;
- import android.os.Bundle;
- import android.os.Environment;
- import android.view.SurfaceHolder;
- import android.view.SurfaceView;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.ImageButton;
-
- public class MainActivity extends Activity{
-
- private SurfaceView surfaceView=null;
- private SurfaceHolder surfaceHolder=null;
- private MediaPlayer media=null;
- private ImageButton btnPlay=null;
- private ImageButton btnStop=null;
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
-
- //獲得surfaceView對象
- surfaceView = (SurfaceView) this.findViewById(R.id.surface);
- //獲得surfaceHolder對象
- surfaceHolder = this.surfaceView.getHolder();
- //設置 SurfaceView類型
- surfaceHolder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS);
-
- //創建MediaPlayer對象
- media=new MediaPlayer();
- try {
- this.media.setDataSource( //設置視頻源,即視頻路徑
- Environment.getExternalStorageDirectory()
- .getPath()
- +"/genwoxue/mybaby.3gp");
- } catch (IllegalArgumentException e) {
- e.printStackTrace();
- } catch (SecurityException e) {
- e.printStackTrace();
- } catch (IllegalStateException e) {
- e.printStackTrace();
- } catch (IOException e) {
- e.printStackTrace();
- }
-
- btnPlay=(ImageButton)super.findViewById(R.id.playvideo);
- btnStop=(ImageButton)super.findViewById(R.id.stopvideo);
-
- //播放視頻
- btnPlay.setOnClickListener(new OnClickListener(){
- @Override
- public void onClick(View v){
- media.setAudioStreamType(AudioManager.STREAM_MUSIC);
- media.setDisplay(MainActivity.this.surfaceHolder);
-
- try {
- media.prepare();
- } catch (IllegalStateException e) {
- e.printStackTrace();
- } catch (IOException e) {
- e.printStackTrace();
- }
- media.start();
- }
-
- });
-
- //停止視頻
- btnStop.setOnClickListener(new OnClickListener(){
- @Override
- public void onClick(View v){
- media.stop();
- }
-
- });
- }
-
- }
三、運行結果
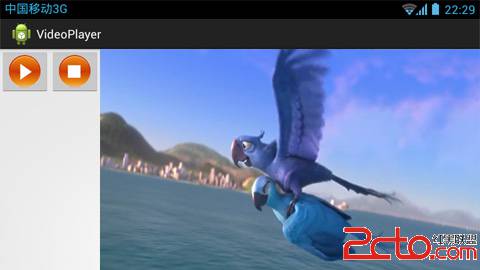
單擊“播放”按鈕,“裡約大冒險”正在為您熱映……
附:
(1)使用其自帶的播放器。指定Action為ACTION_VIEW,Data為Uri,Type為其MIME類型。
[java] view plain copy
- Uri uri = Uri.parse(Environment.getExternalStorageDirectory().getPath()+"/Test_Movie.m4v");
- //調用系統自帶的播放器
- Intent intent = new Intent(Intent.ACTION_VIEW);
- Log.v("URI:::::::::", uri.toString());
- intent.setDataAndType(uri, "video/mp4");
- startActivity(intent);
(2)使用VideoView來播放。在布局文件中使用VideoView結合MediaController來實現對其控制。
[java] view plain copy
- Uri uri = Uri.parse(Environment.getExternalStorageDirectory().getPath()+"/Test_Movie.m4v");
- VideoView videoView = (VideoView)this.findViewById(R.id.video_view);
- videoView.setMediaController(new MediaController(this));
- videoView.setVideoURI(uri);
- videoView.start();
- videoView.requestFocus();