1.使用SharedPreferences處理數據的 新建 儲存 讀取 刪除
SharedPreferences保存後生成的是XML文件,內容是以節點的形勢保存在文件中,SharedPreferences類提供了非常豐富的處理數據的方法下面我向大家介紹一下如何使用SharedPreferences來處理數據。
輸入須要保存的內容
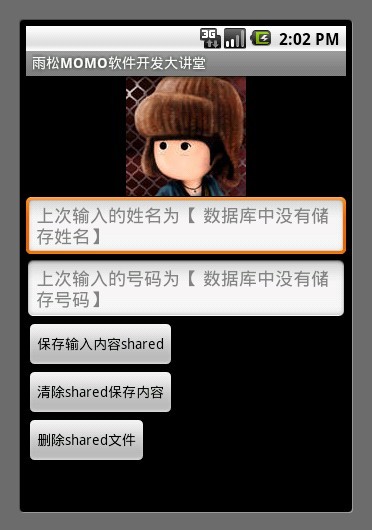
輸入姓名:雨松MOMO
輸入號碼:15810463139
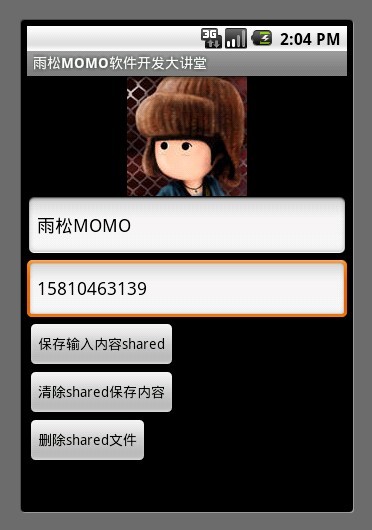
點擊保存成功
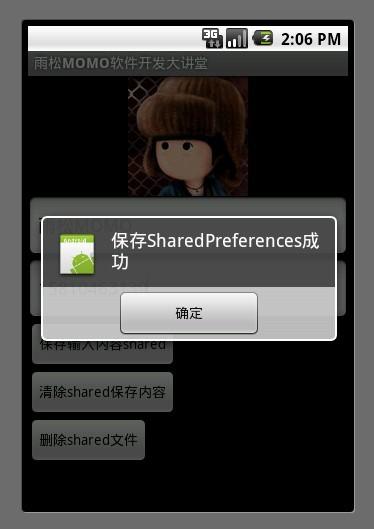
保存成功以後,數據被保存到了data路徑下 /當前包名 (紅框內的包名是我的程序包名) /shared_prefs/main.xml中 , 使用EditPlus 打開保存的內容,我們可以清晰的看到內容是以一個節點一個節點的形式存在XML中。
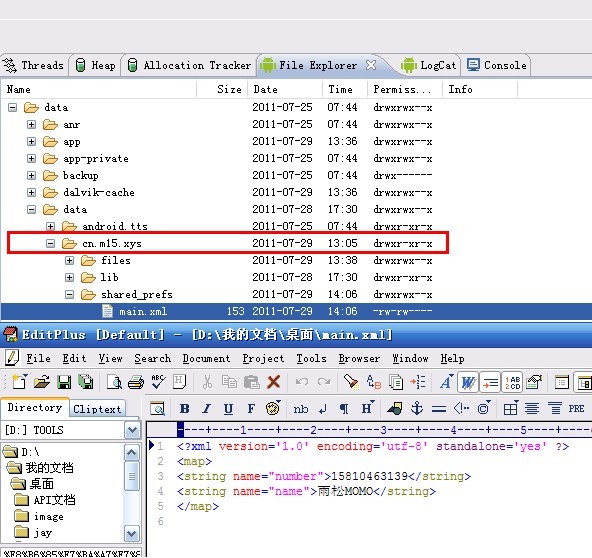
SharedPreferences類中提供了非常方便方法去保存數據與讀取數據大家請看下面的代碼片段,一個程序中可以存在多個SharedPreferences保存的XML文件 ,代碼中只須要根據不同的XML名稱就可以通過方法拿到相應的對象,由於它的批量遍歷查找,當然這樣的作法肯定沒有數據庫更方便快捷,所以在開發中處理一些比較小的零碎的數據就可以保存在這裡,比如說記錄軟件中用戶設置的音量大小,用戶輸入的查找信息等等都可以存在SharedPreferences中。
Java代碼
- public class SPActivity extends Activity {
-
- /**使用SharedPreferences 來儲存與讀取數據**/
- SharedPreferences mShared = null;
-
- /**程序中可以同時存在多個SharedPreferences數據, 根據SharedPreferences的名稱就可以拿到對象**/
- public final static String SHARED_MAIN = "main";
-
- /**SharedPreferences中儲存數據的Key名稱**/
- public final static String KEY_NAME = "name";
- public final static String KEY_NUMBER = "number";
-
- /**SharedPreferences中儲存數據的路徑**/
- public final static String DATA_URL = "/data/data/";
- public final static String SHARED_MAIN_XML = "main.xml";
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- setContentView(R.layout.sharedpreferences);
- /**拿到名稱是SHARED_MAIN 的SharedPreferences對象**/
- mShared = getSharedPreferences(SHARED_MAIN, Context.MODE_PRIVATE);
- /**拿到SharedPreferences中保存的數值 第二個參數為如果SharedPreferences中沒有保存就賦一個默認值**/
- String name = mShared.getString(KEY_NAME, "數據庫中沒有儲存姓名");
- String number = mShared.getString(KEY_NUMBER, "數據庫中沒有儲存號碼");
-
- final EditText editName = (EditText)findViewById(R.id.sp_et0);
- final EditText editNumber = (EditText)findViewById(R.id.sp_et1);
- editName.setHint("上次輸入的姓名為【 " +name+"】");
- editNumber.setHint("上次輸入的號碼為【 " +number+"】");
-
- Button button0 = (Button)findViewById(R.id.sp_button0);
-
- /**監聽按鈕點擊後保存用戶輸入信息到SharedPreferences中**/
- button0.setOnClickListener(new OnClickListener() {
-
- @Override
- public void onClick(View arg0) {
- /**拿到用戶輸入的信息**/
- String name = editName.getText().toString();
- String number = editNumber.getText().toString();
- /**開始保存入SharedPreferences**/
- Editor editor = mShared.edit();
- editor.putString(KEY_NAME, name);
- editor.putString(KEY_NUMBER, number);
- /**put完畢必需要commit()否則無法保存**/
- editor.commit();
- ShowDialog("保存SharedPreferences成功");
-
- }
- });
-
- Button button1 = (Button)findViewById(R.id.sp_button1);
- button1.setOnClickListener(new OnClickListener() {
-
- @Override
- public void onClick(View arg0) {
- /**開始清除SharedPreferences中保存的內容**/
- Editor editor = mShared.edit();
- editor.remove(KEY_NAME);
- editor.remove(KEY_NUMBER);
- //editor.clear();
- editor.commit();
- ShowDialog("清除SharedPreferences數據成功");
- }
- });
-
- Button button2 = (Button)findViewById(R.id.sp_button2);
- button2.setOnClickListener(new OnClickListener() {
-
- @Override
- public void onClick(View arg0) {
- /** 刪除SharedPreferences文件 **/
- File file = new File(DATA_URL + getPackageName().toString()
- + "/shared_prefs", SHARED_MAIN_XML);
- if (file.exists()) {
- file.delete();
- }
- ShowDialog("刪除SharedPreferences文件成功");
- }
- });
-
- super.onCreate(savedInstanceState);
- }
-
- public void ShowDialog(String string) {
- AlertDialog.Builder builder = new AlertDialog.Builder(SPActivity.this);
- builder.setIcon(R.drawable.icon);
- builder.setTitle(string);
- builder.setPositiveButton("確定", new DialogInterface.OnClickListener() {
- public void onClick(DialogInterface dialog, int whichButton) {
- finish();
- }
- });
- builder.show();
- }
- }
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
-
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="vertical"
- >
- <ImageView android:id="@+id/sp_image"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/image"
- android:layout_gravity="center"
- />
- <EditText android:id="@+id/sp_et0"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:hint="請輸入你的姓名">
- </EditText>
- <EditText android:id="@+id/sp_et1"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:hint="請輸入你的號碼">
- </EditText>
- <Button android:id="@+id/sp_button0"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="保存輸入內容shared">
- </Button>
- <Button android:id="@+id/sp_button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="清除shared保存內容">
- </Button>
- <Button android:id="@+id/sp_button2"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="刪除shared文件">
- </Button>
- </LinearLayout>
2.在本地data文件下使用自己生成的文件處理數據的 新建 儲存 讀取 刪除
如果說不想把內容存在SharedPreferences中的話,我們可以自己寫一個文件保存須要的數據,在這裡我將文件保存在系統中的工程路徑下。
輸入需要保存的內容
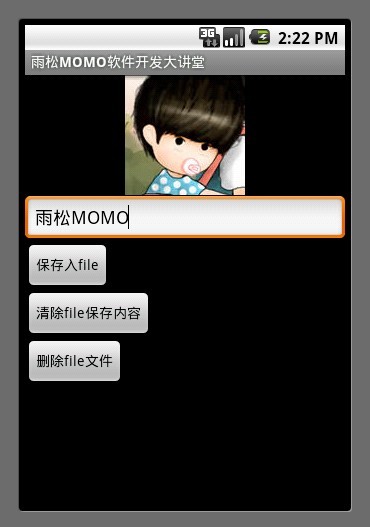
保存完畢後紅框內呈現之前保存的數據
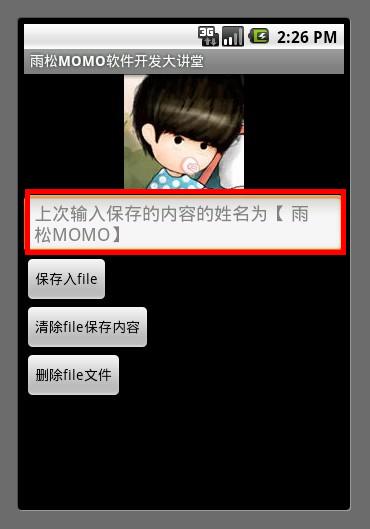
保存文件以後,文件被保存在了當前工程下 files 文件夾的路徑下,這裡說一下data文件夾 如果手機沒有root 權限 用戶是訪問不到的,這種儲存方式有一個麻煩的地方就是文件中保存的數據須要程序員自己去處理 , 好比文件中保存了很多字符串數據 但是我們只須要其中的一部分數據,這樣就須要自己去寫代碼去從文件中拿需要的數據。
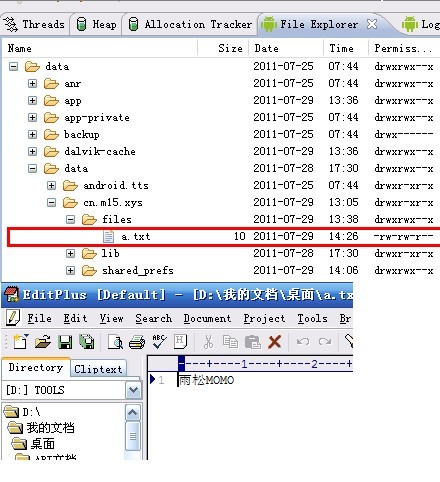
Java代碼
- public class FileActivity extends Activity {
- public final static String FILE_NAME = "a.txt";
-
- /**File中儲存數據的路徑**/
- public final static String DATA_URL = "/data/data/";
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- setContentView(R.layout.file);
- /**讀取內容**/
- String content = loadFile();
- if(content == null) {
- content ="上次沒有輸入內容請輸入";
- }
- String str = "上次輸入保存的內容的姓名為【 " +content + "】";
- final EditText editContent = ((EditText)findViewById(R.id.file_et0));
- editContent.setHint(str);
- Button button0 = (Button)findViewById(R.id.file_button0);
-
- /**監聽按鈕點擊後保存用戶輸入信息到file中**/
- button0.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View arg0) {
- /**拿到用戶輸入的信息**/
- String content = editContent.getText().toString();
- /**開始保存入file**/
- saveFile(content);
- ShowDialog("保存File文件成功");
- }
- });
-
- Button button1 = (Button)findViewById(R.id.file_button1);
- /**監聽按鈕點擊後清空file中內容**/
- button1.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View arg0) {
- cleanFile();
- ShowDialog("清空File文件成功");
- }
- });
-
- Button button2 = (Button)findViewById(R.id.file_button2);
-
- /**監聽按鈕點擊後刪除file文件**/
- button2.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View arg0) {
- File file = new File(DATA_URL + getPackageName().toString()
- + "/files", FILE_NAME);
- if (file.exists()) {
- file.delete();
- }
- ShowDialog("刪除file文件成功");
- }
- });
-
- super.onCreate(savedInstanceState);
- }
-
- /**
- * 保存內容
- * @param str
- */
- public void saveFile(String str) {
- try {
- FileOutputStream outStream = this.openFileOutput(FILE_NAME,
- Context.MODE_WORLD_READABLE);
- outStream.write(str.getBytes());
- outStream.close();
- } catch (FileNotFoundException e) {
- } catch (IOException e) {
- }
- }
-
- /**
- * 因為java刪除文件內容只有一種實現方法,就是把整個文件重寫,只是把須要刪除的那一條記錄去除掉
- */
- public void cleanFile() {
- //如果只須要刪除文件中的一部分內容則須要在這裡對字符串做一些操作
- String cleanStr = "";
- try {
- FileOutputStream outStream = this.openFileOutput(FILE_NAME,
- Context.MODE_WORLD_READABLE);
- outStream.write(cleanStr.getBytes());
- outStream.close();
- } catch (FileNotFoundException e) {
- } catch (IOException e) {
- }
-
- }
-
- public String loadFile() {
- try {
- FileInputStream inStream = this.openFileInput(FILE_NAME);
- ByteArrayOutputStream stream = new ByteArrayOutputStream();
- byte[] buffer = new byte[1024];
- int length = -1;
- while ((length = inStream.read(buffer)) != -1) {
- stream.write(buffer, 0, length);
- }
- stream.close();
- inStream.close();
- return stream.toString();
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- } catch (IOException e) {
- }
- return null;
- }
-
- public void ShowDialog(String str) {
- AlertDialog.Builder builder = new AlertDialog.Builder(FileActivity.this);
- builder.setIcon(R.drawable.icon);
- builder.setTitle(str);
- builder.setPositiveButton("確定", new DialogInterface.OnClickListener() {
- public void onClick(DialogInterface dialog, int whichButton) {
- finish();
- }
- });
- builder.show();
- }
- }
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
-
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="vertical"
- >
- <ImageView android:id="@+id/file_image"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/jay"
- android:layout_gravity="center"
- />
-
- <EditText android:id="@+id/file_et0"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:hint="請輸入需要保存的內容">
- </EditText>
- <Button android:id="@+id/file_button0"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="保存入file">
- </Button>
- <Button android:id="@+id/file_button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="清除file保存內容">
- </Button>
- <Button android:id="@+id/file_button2"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="刪除file文件">
- </Button>
- </LinearLayout>
3.在本地程序res/raw中讀取數據操作
Android 下提供了專門讀取程序res/raw路徑下資源的方法,但是沒有提供寫入raw內容的方法,也就是說只能讀不能寫,在做軟件的時候有時須要讀取大量的文字資源,由於這些資源文字在軟件中不會改變所以無需去對它的內容重寫修改,就可以使用raw來操作數據。
如圖所示:在列表中讀取.bin文件中的內容分別顯示在listView中
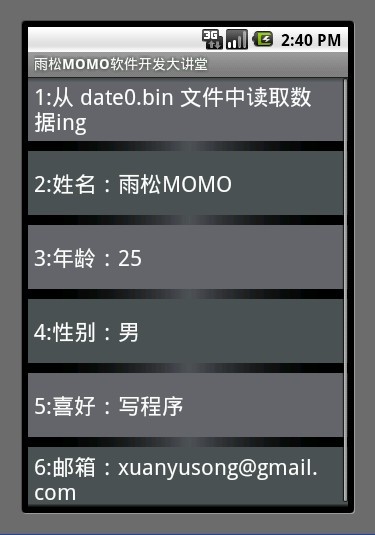
如圖所示在raw路徑下存了一個文件date0.bin ,下面是bin文件中保存的內容,程序中須要對這個.bin文件的內容進行讀取並顯示在屏幕中。
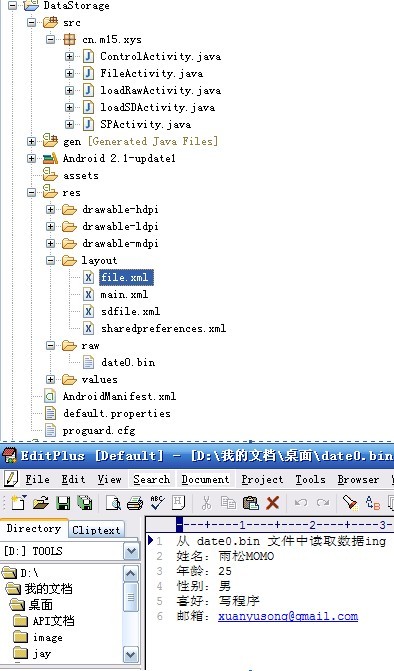
下面給出代碼的實現
Java代碼
- public class loadRawActivity extends ListActivity {
-
- private class MyListAdapter extends BaseAdapter {
- private int[] colors = new int[] { 0xff626569, 0xff4f5257 };
-
- public MyListAdapter(Context context) {
- mContext = context;
- }
-
- public int getCount() {
- return inpormation.length;
- }
-
- @Override
- public boolean areAllItemsEnabled() {
- return false;
- }
-
- public Object getItem(int position) {
- return position;
- }
-
- public long getItemId(int position) {
- return position;
- }
-
- public View getView(int position, View convertView, ViewGroup parent) {
- TextView tv;
- if (convertView == null) {
- tv = (TextView) LayoutInflater.from(mContext).inflate(
- android.R.layout.simple_list_item_1, parent, false);
- } else {
- tv = (TextView) convertView;
- }
- int colorPos = position % colors.length;
- tv.setBackgroundColor(colors[colorPos]);
- tv.setText(String.valueOf(position + 1) + ":"
- + inpormation[position]);
- return tv;
- }
-
- private Context mContext;
- }
-
- String[] inpormation = null;
- ListView listView;
-
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- readFile(R.raw.date0);
- setListAdapter(new MyListAdapter(this));
- listView = getListView();
- int[] colors = { 0, 0xFF505259, 0 };
- listView
- .setDivider(new GradientDrawable(Orientation.RIGHT_LEFT, colors));
- listView.setDividerHeight(10);
- super.onCreate(savedInstanceState);
- }
-
- /**
- * 從raw中讀取數據
- * @param ID
- */
- public void readFile(int ID) {
- InputStream in = null;
- String temp = "";
- try {
- in = this.getResources().openRawResource(ID);
- byte[] buff = new byte[1024];// 緩存
- int rd = 0;
- ByteArrayOutputStream baos = new ByteArrayOutputStream();
- while ((rd = in.read(buff)) != -1) {
- baos.write(buff, 0, rd);
- temp = new String(baos.toByteArray(), "UTF-8");
- }
- baos.close();
- in.close();
- inpormation = temp.split("\r\n");
- } catch (Exception e) {
- Toast.makeText(this, "文件沒有找到", 2000).show();
- }
- }
-
- }
4.在SD卡中處理新建 寫入 讀取 刪除 的操作
可以把數據保存在SD卡中,在SD卡中建立一個文件去保存數據,這裡說一下 ,SD卡 用戶是可以訪問的,也就是說可以把一些可有可無的數據存在SD卡中,即使用戶刪除了卡中的內容也不會影像軟件的使用。
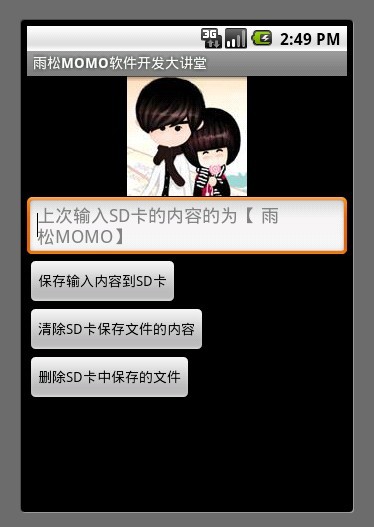
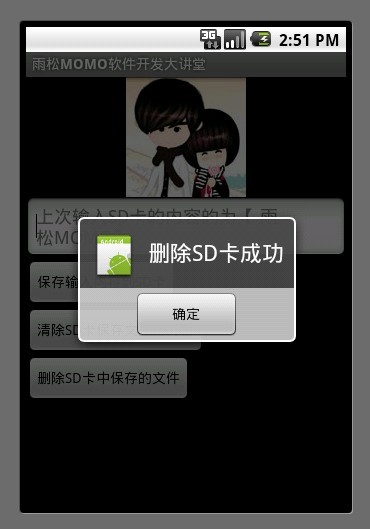
Java代碼
- public class loadSDActivity extends Activity {
- public final static String FILE_NAME = "b.txt";
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- setContentView(R.layout.sdfile);
- /**讀取內容**/
- String content = loadFile();
- if(content == null) {
- content ="上次沒有輸入內容請輸入";
- }
-
- final EditText editContent = (EditText)findViewById(R.id.sdfile_et0);
- editContent.setHint("上次輸入SD卡的內容的為【 " +content + "】");
- Button button0 = (Button)findViewById(R.id.sdfile_button0);
-
- /**監聽按鈕點擊後保存用戶輸入信息到SD卡中**/
- button0.setOnClickListener(new OnClickListener() {
-
- @Override
- public void onClick(View arg0) {
- /**拿到用戶輸入的信息**/
- String content = editContent.getText().toString();
- /**開始保存入SD卡**/
- saveFile(content);
- ShowDialog("保存SD卡文件成功");
- }
- });
- Button button1 = (Button)findViewById(R.id.sdfile_button1);
-
- /**去清除SD卡保存的內容**/
- button1.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View arg0) {
- cleanFile();
- ShowDialog("清除SD卡文件中的內容成功");
- }
- });
- Button button2 = (Button)findViewById(R.id.sdfile_button2);
-
- /**刪除SD卡保存的文件**/
- button2.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View arg0) {
- DeleteSDFile();
- }
- });
-
- super.onCreate(savedInstanceState);
- }
-
- /**
- * 保存入SD卡中
- * @param str
- */
- public void saveFile(String str) {
- FileOutputStream fileOutputStream = null;
-
- File file = new File(Environment.getExternalStorageDirectory(),
- FILE_NAME);
- try {
- fileOutputStream = new FileOutputStream(file);
- fileOutputStream.write(str.getBytes());
- fileOutputStream.close();
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- }catch (IOException e) {
- e.printStackTrace();
- }
- }
-
- /**
- * 讀取SD卡的內容
- * @return
- */
- public String loadFile() {
- String path = Environment.getExternalStorageDirectory() +"/" + FILE_NAME;
- try {
-
- FileInputStream fi = new FileInputStream(path);
- BufferedReader br = new BufferedReader(new InputStreamReader(
- fi));
- String readString = new String();
- while ((readString = br.readLine()) != null) {
- //數據多的話須要在這裡處理 readString
- return readString;
- }
- fi.close();
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- } catch (IOException e) {
- e.printStackTrace();
- }
-
- return null;
- }
-
- /**
- * 刪除SD卡
- */
- public void DeleteSDFile() {
- String path = Environment.getExternalStorageDirectory() + "/"
- + FILE_NAME;
- File file1 = new File(path);
- boolean isdelte = file1.delete();
- if(isdelte) {
- ShowDialog("刪除SD卡成功");
- }else {
- finish();
- }
- }
-
- /**
- * 因為java刪除文件內容只有一種實現方法,就是把整個文件重寫,只是把須要刪除的那一條記錄去除掉
- */
- public void cleanFile() {
- //如果只須要刪除文件中的一部分內容則須要在這裡對字符串做一些操作
- String cleanStr = "";
- FileOutputStream fileOutputStream = null;
-
- File file = new File(Environment.getExternalStorageDirectory(),
- FILE_NAME);
- try {
- fileOutputStream = new FileOutputStream(file);
- fileOutputStream.write(cleanStr.getBytes());
- fileOutputStream.close();
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- }catch (IOException e) {
- e.printStackTrace();
- }
- }
- public void ShowDialog(String str) {
- AlertDialog.Builder builder = new AlertDialog.Builder(loadSDActivity.this);
- builder.setIcon(R.drawable.icon);
- builder.setTitle(str);
- builder.setPositiveButton("確定", new DialogInterface.OnClickListener() {
- public void onClick(DialogInterface dialog, int whichButton) {
- finish();
- }
- });
- builder.show();
- }
- }
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
-
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="vertical"
- >
- <ImageView android:id="@+id/sdfile_image"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/g"
- android:layout_gravity="center"
- />
- <EditText android:id="@+id/sdfile_et0"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:hint="請輸入需要保存到SD卡的內容">
- </EditText>
- <Button android:id="@+id/sdfile_button0"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="保存輸入內容到SD卡">
- </Button>
- <Button android:id="@+id/sdfile_button1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="清除SD卡保存文件的內容">
- </Button>
- <Button android:id="@+id/sdfile_button2"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:text="刪除SD卡中保存的文件">
- </Button>
- </LinearLayout>
源碼下載地址:http://vdisk.weibo.com/s/a9n91