Android系統在數據存儲和數據共享方面提供了多種方式,包括前面我們講過的使用SQLite數據庫的方式,本文將為大家講解另一種共享數據和存儲數據的方式-共享參數類SharedPreferences的使用。
一、SharedPreferences簡介
SharedPreferences是一種輕量級的數據存儲方式,學過Web開發的同學,可以想象它是一個小小的Cookie。它可以用鍵值對的方式把簡單數據類型(boolean、int、float、long和String)存儲在應用程序的私有目錄下(data/data/包名/shared_prefs/)自己定義的xml文件中。下面我們用一個記錄音樂播放進度的例子來學習SharedPreferences的使用。
二、SharedPreferences實例
1、建立一個新的項目Lesson19_HelloSharedPreferences,Activity名字叫MainHelloSharedPreferences.java。
2、建立一個MusicService.java的Service,代碼如下:
Java代碼
- package android.basic.lesson19;
-
- import android.app.Service;
- import android.content.Context;
- import android.content.Intent;
- import android.content.SharedPreferences;
- import android.media.MediaPlayer;
- import android.os.IBinder;
- import android.widget.Toast;
-
- public class MusicService extends Service {
-
- //定義MediaPlayer播放器變量
- MediaPlayer mPlayer = new MediaPlayer();
-
- @Override
- public void onCreate() {
- Toast.makeText(getApplicationContext(), "Service onCreate()", Toast.LENGTH_LONG).show();
- //創建播放器
- mPlayer = MediaPlayer.create(getApplicationContext(), R.raw.babayetu);
- //設置自動循環
- mPlayer.setLooping(true);
- }
-
- @Override
- public IBinder onBind(Intent intent) {
- Toast.makeText(getApplicationContext(), "Service onBind()", Toast.LENGTH_LONG).show();
- //獲得SharedPreferences對象
- SharedPreferences preferences = this.getSharedPreferences("MusicCurrentPosition",Context.MODE_WORLD_WRITEABLE);
- //播放器跳轉到上一次播放的進度
- mPlayer.seekTo(preferences.getInt("CurrentPosition", 0));
- //開始播放
- mPlayer.start();
- return null;
- }
-
- @Override
- public boolean onUnbind(Intent intent){
- Toast.makeText(getApplicationContext(), "Service onUnbind()", Toast.LENGTH_LONG).show();
- //獲得SharedPreferences對象
- SharedPreferences preferences = this.getSharedPreferences("MusicCurrentPosition",Context.MODE_WORLD_WRITEABLE);
- Toast.makeText(getApplicationContext(), "CurrentPosition="+mPlayer.getCurrentPosition(), Toast.LENGTH_LONG).show();
- //獲得editor對象,寫入一個整數到SharePreferences中,記住要用commit()提交,否則不會實現寫入操作
- preferences.edit().putInt("CurrentPosition",mPlayer.getCurrentPosition()).commit();
- mPlayer.stop();
- return false;
- }
- }
3、更改AndroidManifest.xml內容如下:
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
- <manifest android:versionname="1.0" android:versioncode="1" xmlns:android="http://schemas.android.com/apk/res/android" package="android.basic.lesson19">
- <application android:icon="@drawable/icon" android:label="@string/app_name">
- <activity android:label="@string/app_name" android:name=".MainHelloSharedPreferences">
- <intent -filter="">
- <action android:name="android.intent.action.MAIN">
- <category android:name="android.intent.category.LAUNCHER">
- </category></action></intent>
- </activity>
- <service android:name=".MusicService" android:enabled="true">
- </service>
- </application>
- <uses android:minsdkversion="8" -sdk="">
-
- </uses></manifest>
4、res/layout/mail.xml的內容如下:
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
- <linearlayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent">
- <textview android:layout_width="wrap_content" android:layout_height="wrap_content" android:textsize="20sp" android:text="SharedPreferences的使用" android:id="@+id/TextView01">
- </textview>
-
- <button android:layout_width="wrap_content" android:layout_height="wrap_content" android:textsize="20sp" android:text="綁定音樂播放服務" android:id="@+id/Button01" android:layout_margintop="10dp">
- </button>
- <button android:layout_width="wrap_content" android:layout_height="wrap_content" android:textsize="20sp" android:text="解綁定音樂播放服務" android:id="@+id/Button02" android:layout_margintop="10dp">
- </button>
- </linearlayout>
5、MainHelloSharedPreferences.java的內容如下:
Java代碼
- package android.basic.lesson19;
-
- import android.app.Activity;
- import android.content.ComponentName;
- import android.content.Context;
- import android.content.Intent;
- import android.content.ServiceConnection;
- import android.os.Bundle;
- import android.os.IBinder;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.Toast;
-
- public class MainHelloSharedPreferences extends Activity {
- /** Called when the activity is first created. */
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
-
- //定義UI組件
- Button b1 = (Button) findViewById(R.id.Button01);
- Button b2 = (Button) findViewById(R.id.Button02);
-
- //定義ServiceConnection對象
- final ServiceConnection conn = new ServiceConnection() {
-
- @Override
- public void onServiceConnected(ComponentName name, IBinder service) {
- }
-
- @Override
- public void onServiceDisconnected(ComponentName name) {
- }
- };
-
- //定義按鈕的單擊監聽器
- OnClickListener ocl = new OnClickListener() {
- @Override
- public void onClick(View v) {
- Intent intent = new Intent(MainHelloSharedPreferences.this,
- android.basic.lesson19.MusicService.class);
- switch (v.getId()) {
- case R.id.Button01:
- Toast.makeText(getApplicationContext(), "Button01 onClick", Toast.LENGTH_LONG).show();
- //綁定服務
- bindService(intent,conn,Context.BIND_AUTO_CREATE);
- break;
- case R.id.Button02:
- Toast.makeText(getApplicationContext(), "Button02 onClick", Toast.LENGTH_LONG).show();
- //取消綁定
- unbindService(conn);
- break;
- }
- }
- };
-
- //綁定單擊監聽器
- b1.setOnClickListener(ocl);
- b2.setOnClickListener(ocl);
-
- }
- }
6、運行程序,查看運行情況:
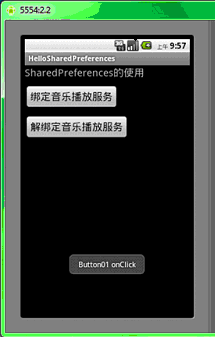
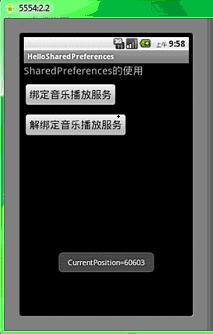
查看File Explorer,在/data/data/android.basic.lesson19/shared_prefs/目錄下有一個MusicCurrentPosition.xml文件,點擊右上角的按鈕pull a file from the device,可以把這個xml文拷貝出來:
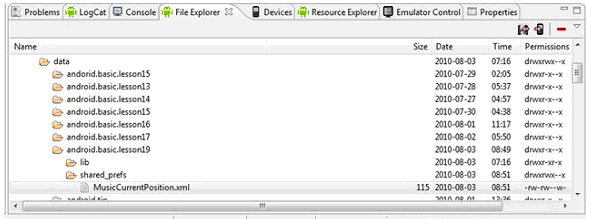
7、查看MusicCurrentPosition.xml的內容,可以看到音樂播放進度的數據存貯在這個xml中:
XML/HTML代碼
- <?xml version='1.0' encoding='utf-8' standalone='yes' ?>
- <map>
- <int name="CurrentPosition" value="15177">
- </int></map>
大家可以試著擴展下本實例,為Activity添加一個按鈕,點擊它實現的功能是取出SharedPreferences中的播放進度數據,顯示在另一個文本框Textview02裡。下面直接貼上最終的運行效果圖,至於程序,大家可以試著自己寫一下,更加深刻的理解Share,同一個進程中的不同組件是否都可以訪問此共享的持久化數據?這一點是不是與Cookie有些類似?
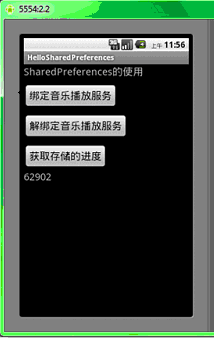