上一節講到了Gallery畫廊,本節主要講解Android組件Service,主要內容包括:Service的概念、生命周期及其應用實例。
一、Service簡介
Service是Android程序中四大基礎組件之一,它和Activity一樣都是Context的子類,只不過它沒有UI界面,是在後台運行的組件。
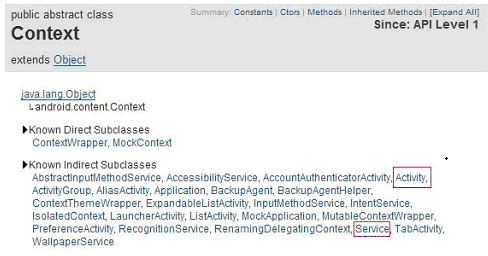
二、Service的生命周期
Service對象不能自己啟動,需要通過某個Activity、Service或者其他Context對象來啟動。啟動的方法有兩種,Context.startService和Context.bindService()。兩種方式的生命周期是不同的,具體如下所示。
Context.startService方式的生命周期:
啟動時,startService –> onCreate() –> onStart()
停止時,stopService –> onDestroy()
Context.bindService方式的生命周期:
綁定時,bindService -> onCreate() –> onBind()
解綁定時,unbindService –>onUnbind() –> onDestory()
三、Service應用實例:控制音樂播放的Service
下面我們用一個可以控制在後台播放音樂的例子來演示剛才所學知識,同學們可以通過該例子可以明顯看到通過綁定方式運行的Service在綁定對象被銷毀後也被銷毀了。
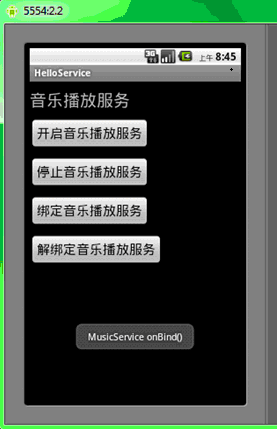
下面是編寫該實例的步驟:
1、建立一個新項目名字叫Lesson14_HelloService,Activity起名叫MainHelloService.java。
2、res/layout/main.xml中代碼如下:
XML/HTML代碼
- < ?xml version="1.0" encoding="utf-8"?>
- <linearlayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent">
- <textview android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="音樂播放服務" android:textsize="25sp" android:layout_margintop="10dp">
- <button android:text="開啟音樂播放服務" android:textsize="20sp" android:id="@+id/Button01" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margintop="10dp">
- </button>
- <button android:text="停止音樂播放服務" android:textsize="20sp" android:id="@+id/Button02" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margintop="10dp">
- </button>
-
- <button android:text="綁定音樂播放服務" android:textsize="20sp" android:id="@+id/Button03" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margintop="10dp">
- </button>
- <button android:text="解綁定音樂播放服務" android:textsize="20sp" android:id="@+id/Button04" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margintop="10dp">
- </button>
- </textview></linearlayout>
3、在res目錄中建立一個raw目錄,並把一個音樂文件babayetu.mp3拷貝進來。
4、在Activity的同目錄新建一個service文件MusicService.java。
Java代碼
- package android.basic.lesson14;
-
- import android.app.Service;
- import android.content.Intent;
- import android.media.MediaPlayer;
- import android.os.IBinder;
- import android.util.Log;
- import android.widget.Toast;
-
- public class MusicService extends Service {
-
- //為日志工具設置標簽
- String tag ="MusicService";
-
- //定義音樂播放器變量
- MediaPlayer mPlayer;
-
- //其他對象通過bindService方法通知該Service時該方法會被調用
- @Override
- public IBinder onBind(Intent intent) {
- Toast.makeText(this,"MusicService onBind()",Toast.LENGTH_SHORT).show();
- Log.i(tag, "MusicService onBind()");
- mPlayer.start();
- return null;
- }
-
- //其他對象通過unbindService方法通知該Service時該方法會被調用
- @Override
- public boolean onUnbind(Intent intent){
- Toast.makeText(this, "MusicService onUnbind()", Toast.LENGTH_SHORT).show();
- Log.i(tag, "MusicService onUnbind()");
- mPlayer.stop();
- return false;
- }
-
- //該服務不存在需要被創建時被調用,不管startService()還是bindService()都會在啟動時調用該方法
- @Override
- public void onCreate(){
- Toast.makeText(this, "MusicService onCreate()", Toast.LENGTH_SHORT).show();
- //創建一個音樂播放器對象
- mPlayer=MediaPlayer.create(getApplicationContext(), R.raw.babayetu);
- //設置可以重復播放
- mPlayer.setLooping(true);
- Log.i(tag, "MusicService onCreate()");
- }
-
- //用startService方法調用該服務時,在onCreate()方法調用之後,會調用改方法
- @Override
- public void onStart(Intent intent,int startid){
- Toast.makeText(this,"MusicService onStart",Toast.LENGTH_SHORT).show();
- Log.i(tag, "MusicService onStart()");
- mPlayer.start();
- }
-
- //該服務被銷毀時調用該方法
- @Override
- public void onDestroy(){
- Toast.makeText(this, "MusicService onDestroy()", Toast.LENGTH_SHORT).show();
- mPlayer.stop();
- Log.i(tag, "MusicService onDestroy()");
- }
- }
5、MainHelloService.java中的代碼:
Java代碼
- package android.basic.lesson14;
-
- import android.app.Activity;
- import android.content.ComponentName;
- import android.content.Context;
- import android.content.Intent;
- import android.content.ServiceConnection;
- import android.os.Bundle;
- import android.os.IBinder;
- import android.util.Log;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- import android.widget.Toast;
-
- public class MainHelloService extends Activity {
-
- //為日志工具設置標簽
- String tag = "MusicService";
-
- /** Called when the activity is first created. */
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
-
- //輸出Toast消息和日志記錄
- Toast.makeText(MainHelloService.this, "MainHelloService onCreate", Toast.LENGTH_SHORT).show();
- Log.i(tag, "MainHelloService onCreate");
-
- //定義組件對象
- Button b1= (Button)findViewById(R.id.Button01);
- Button b2= (Button)findViewById(R.id.Button02);
- Button b3= (Button)findViewById(R.id.Button03);
- Button b4= (Button)findViewById(R.id.Button04);
-
- //定義服務鏈接對象
- final ServiceConnection conn = new ServiceConnection(){
-
- @Override
- public void onServiceConnected(ComponentName name, IBinder service) {
- Toast.makeText(MainHelloService.this, "ServiceConnection onServiceConnected", Toast.LENGTH_SHORT).show();
- Log.i(tag, "ServiceConnection onServiceConnected");
-
- }
-
- @Override
- public void onServiceDisconnected(ComponentName name) {
- Toast.makeText(MainHelloService.this, "ServiceConnection onServiceDisconnected", Toast.LENGTH_SHORT).show();
- Log.i(tag, "ServiceConnection onServiceDisconnected");
-
- }};
-
- //定義點擊監聽器
- OnClickListener ocl= new OnClickListener(){
-
- @Override
- public void onClick(View v) {
- //顯示指定intent所指的對象是個Service
- Intent intent = new Intent(MainHelloService.this,android.basic.lesson14.MusicService.class);
- switch(v.getId()){
- case R.id.Button01:
- //開始服務
- startService(intent);
- break;
- case R.id.Button02:
- //停止服務
- stopService(intent);
- break;
- case R.id.Button03:
- //綁定服務
- bindService(intent,conn,Context.BIND_AUTO_CREATE);
- break;
- case R.id.Button04:
- //解除綁定
- unbindService(conn);
- break;
- }
- }
- };
-
- //綁定點擊監聽器
- b1.setOnClickListener(ocl);
- b2.setOnClickListener(ocl);
- b3.setOnClickListener(ocl);
- b4.setOnClickListener(ocl);
-
- }
-
- @Override
- public void onDestroy(){
- super.onDestroy();
- Toast.makeText(MainHelloService.this, "MainHelloService onDestroy", Toast.LENGTH_SHORT).show();
- Log.i(tag, "MainHelloService onDestroy");
- }
- }
了解了Service的概念、生命周期,又跟著做了一個實例,相信大家現在對Service的使用已經有了一個基本的掌握了。