android通訊錄導航欄源碼(一),android導航欄
通訊錄導航欄源碼:
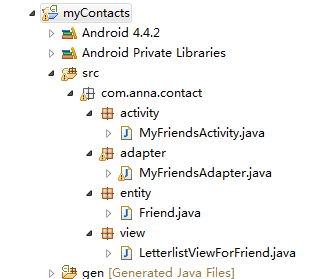
1.activity

![]()
1 package com.anna.contact.activity;
2
3 import java.util.ArrayList;
4 import java.util.HashMap;
5 import java.util.List;
6
7 import com.anna.contact.R;
8 import com.anna.contact.adapter.MyFriendsAdapter;
9 import com.anna.contact.entity.Friend;
10 import com.anna.contact.view.LetterlistViewForFriend;
11 import com.anna.contact.view.LetterlistViewForFriend.OnTouchingLetterChangedListener;
12 import com.iflytek.voiceads.AdError;
13 import com.iflytek.voiceads.AdKeys;
14 import com.iflytek.voiceads.IFLYAdListener;
15 import com.iflytek.voiceads.IFLYAdSize;
16 import com.iflytek.voiceads.IFLYFullScreenAd;
17
18 import android.app.Activity;
19 import android.content.Context;
20 import android.graphics.PixelFormat;
21 import android.os.Bundle;
22 import android.os.Handler;
23 import android.view.LayoutInflater;
24 import android.view.View;
25 import android.view.Window;
26 import android.view.WindowManager;
27 import android.view.ViewGroup.LayoutParams;
28 import android.widget.AdapterView;
29 import android.widget.AdapterView.OnItemClickListener;
30 import android.widget.ListView;
31 import android.widget.TextView;
32 import android.widget.Toast;
33
34 public class MyFriendsActivity extends Activity {
35 private IFLYFullScreenAd ad;
36
37 ListView lv_friend;
38 String[] strings;// 存放存在的漢語拼音首字母
39 List<Friend> friends;
40 //右邊字母導航
41 LetterlistViewForFriend letterlistViewForFriend;
42 HashMap<String, Integer> alphaIndex;// 存放存在的漢語拼音首字母和與之對應的列表位置
43 TextView overlay;
44 Handler handler;
45 OverlayThread overlayThread; // 隱藏字母提示框
46
47 @Override
48 protected void onCreate(Bundle savedInstanceState) {
49 // TODO Auto-generated method stub
50 super.onCreate(savedInstanceState);
51 requestWindowFeature(Window.FEATURE_NO_TITLE);
52 createAd();
53 setContentView(R.layout.vip_myactivity_myfriend);
54 overlayThread = new OverlayThread();
55 handler = new Handler();
56 /* 初始化右邊導航 */
57 initOverlay();
58 /* 初始化通訊錄 */
59 initData();
60 lv_friend = (ListView) this.findViewById(R.id.vip_myfriend_lv_1);
61
62 lv_friend.setAdapter(new MyFriendsAdapter(MyFriendsActivity.this,
63 friends));
64 lv_friend.setOnItemClickListener(new OnItemClickListener() {
65
66 @Override
67 public void onItemClick(AdapterView<?> arg0, View arg1, int arg2,
68 long arg3) {
69 // TODO Auto-generated method stub
70
71 }
72
73 });
74
75 letterlistViewForFriend=(LetterlistViewForFriend)this.findViewById(R.id.LetterlistViewForFriend);
76 letterlistViewForFriend.setOnTouchingLetterChangedListener(new OnTouchingLetterChangedListener() {
77
78 @Override
79 public void onTouchingLetterChanged(String s) {
80 // TODO Auto-generated method stub
81 System.out.println(s);
82 int position = alphaIndex.get(s);
83 if (alphaIndex.get(s) != null) {
84 lv_friend.setSelection(position);
85 }
86 overlay.setText(s);
87 overlay.setVisibility(View.VISIBLE);
88 handler.removeCallbacks(overlayThread);
89 // 延遲一秒後執行,讓overlay為不可見
90 handler.postDelayed(overlayThread, 1500);
91 }
92 });
93
94 }
95 // 初始化漢語拼音首字母彈出提示框
96 private void initOverlay() {
97 LayoutInflater inflater = LayoutInflater.from(this);
98 overlay = (TextView) inflater.inflate(R.layout.overlay_layout, null);
99 overlay.setVisibility(View.INVISIBLE);
100 WindowManager.LayoutParams lp = new WindowManager.LayoutParams(
101 LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT,
102 WindowManager.LayoutParams.TYPE_APPLICATION,
103 WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE
104 | WindowManager.LayoutParams.FLAG_NOT_TOUCHABLE,
105 PixelFormat.TRANSLUCENT);
106 WindowManager windowManager = (WindowManager) this
107 .getSystemService(Context.WINDOW_SERVICE);
108 windowManager.addView(overlay, lp);
109 }
110
111 public class OverlayThread implements Runnable {
112
113 @Override
114 public void run() {
115 // TODO Auto-generated method stub
116 overlay.setVisibility(View.INVISIBLE);
117 }
118
119 }
120
121 private void initData() {
122 List<String> list_data = new ArrayList<String>();
123 list_data.add("Alex");
124 list_data.add("Anna");
125 list_data.add("Blic");
126 list_data.add("Blpha");
127 list_data.add("Clex");
128 list_data.add("Clic");
129 list_data.add("Clpha");
130 list_data.add("Clex");
131 list_data.add("Dlic");
132 list_data.add("Dlpha");
133 list_data.add("Dace");
134 list_data.add("Dlpha");
135 list_data.add("Elic");
136 list_data.add("Elpha");
137 list_data.add("Eace");
138 list_data.add("Elpha");
139 list_data.add("Elea");
140 list_data.add("Flpha");
141 list_data.add("Flea");
142 list_data.add("Glpha");
143 list_data.add("Gace");
144 list_data.add("Glpha");
145 list_data.add("Hlea");
146 list_data.add("Ilpha");
147 list_data.add("Jace");
148 list_data.add("Jlpha");
149 list_data.add("Jenney");
150 list_data.add("Kitty");
151 list_data.add("Klpha");
152 list_data.add("Kath");
153 list_data.add("Lace");
154 list_data.add("Llpha");
155 list_data.add("Moon");
156 list_data.add("Merry");
157 list_data.add("Nice");
158 list_data.add("Object");
159 list_data.add("Purplegrape");
160 list_data.add("Queen");
161 list_data.add("Qearl");
162 list_data.add("Rose");
163 list_data.add("Rain");
164 list_data.add("Sunny");
165 list_data.add("Sweet");
166 list_data.add("Tiger");
167 list_data.add("Ternence");
168 list_data.add("Uea");
169 list_data.add("Uea");
170 list_data.add("Wendy");
171 list_data.add("Winnie");
172 list_data.add("Vivian");
173 list_data.add("Vicky");
174 list_data.add("Vanessa");
175 list_data.add("Yvette");
176 list_data.add("Yolanda");
177 list_data.add("Yvonne");
178 list_data.add("Zoey");
179
180 friends = new ArrayList<Friend>();
181 alphaIndex = new HashMap<String, Integer>();
182
183 for (int i = 0; i < list_data.size(); i++) {
184 Friend friend = new Friend();
185 friend.setName(list_data.get(i));
186 friends.add(friend);
187 }
188 strings = new String[friends.size()];
189 for (int i = 0; i < friends.size(); i++) {
190 char letter = friends.get(i).getName().substring(0, 1).charAt(0);
191 String currentLetter = (letter + "").toUpperCase();
192 // 上一個漢語拼音首字母,如果不存在為""
193 String lastLetter = (i - 1) > 0 ? (friends.get(i - 1).getName()
194 .substring(0, 1).charAt(0) + "").toUpperCase() : "";
195 if (!lastLetter.equals(currentLetter)) {
196 alphaIndex.put(currentLetter, i);
197 strings[i]=currentLetter;
198 }
199
200 }
201
202 }
203
204
205 private void createAd() {
206 ad = IFLYFullScreenAd.createFullScreenAd(this,
207 "FDDE8F2023143C2E8702E80CE88B97CA");
208 ad.setAdSize(IFLYAdSize.FULLSCREEN);
209 ad.setParameter(AdKeys.SHOW_TIME_FULLSCREEN, "6000");
210 ad.setParameter(AdKeys.DOWNLOAD_ALERT, "true");
211 ad.loadAd(new IFLYAdListener() {
212
213 @Override
214 public void onAdReceive() {
215 ad.showAd();
216 }
217
218 @Override
219 public void onAdFailed(AdError arg0) {
220 Toast.makeText(
221 getApplicationContext(),
222 arg0.getErrorCode() + "****"
223 + arg0.getErrorDescription(), 0).show();
224
225 }
226
227
228 @Override
229 public void onAdClose() {
230
231 }
232
233 @Override
234 public void onAdClick() {
235
236 }
237
238 @Override
239 public void onAdExposure() {
240 // TODO Auto-generated method stub
241
242 }
243 });
244 }
245
246 @Override
247 protected void onDestroy() {
248 // TODO Auto-generated method stub
249 super.onDestroy();
250 WindowManager windowManager = (WindowManager) this
251 .getSystemService(Context.WINDOW_SERVICE);
252 windowManager.removeView(overlay);
253 }
254
255 }
View Code
2.Adapter

![]()
1 package com.anna.contact.adapter;
2
3 import java.util.List;
4
5 import com.anna.contact.R;
6 import com.anna.contact.entity.Friend;
7
8 import android.content.Context;
9 import android.view.LayoutInflater;
10 import android.view.View;
11 import android.view.ViewGroup;
12 import android.widget.BaseAdapter;
13 import android.widget.ImageView;
14 import android.widget.TextView;
15
16 public class MyFriendsAdapter extends BaseAdapter {
17 LayoutInflater inflater;
18 List<Friend> list;
19
20 public MyFriendsAdapter(Context context, List<Friend> list) {
21 inflater = LayoutInflater.from(context);
22 this.list = list;
23 }
24
25 @Override
26 public int getCount() {
27 // TODO Auto-generated method stub
28 return list.size();
29 }
30
31 @Override
32 public Object getItem(int position) {
33 // TODO Auto-generated method stub
34 return list.get(position);
35 }
36
37 @Override
38 public long getItemId(int position) {
39 // TODO Auto-generated method stub
40 return position;
41 }
42
43 @SuppressWarnings("null")
44 @Override
45 public View getView(int position, View convertView, ViewGroup parent) {
46 // TODO Auto-generated method stub
47 viewHolder holder;
48 if (convertView == null) {
49 convertView = inflater.inflate(
50 R.layout.vip_myactivity_myfriend_item, null);
51 holder = new viewHolder();
52 holder.vip_friend_letter = (TextView) convertView
53 .findViewById(R.id.vip_friend_letter);
54 holder.vip_friend_name = (TextView) convertView
55 .findViewById(R.id.vip_friend_item_name);
56 convertView.setTag(holder);
57 } else {
58 holder = (viewHolder) convertView.getTag();
59 }
60
61 holder.vip_friend_name.setText(list.get(position).getName());
62 char letter = list.get(position).getName().substring(0, 1).charAt(0);
63 String currentLetter = (letter + "").toUpperCase();
64
65 String lastLetter = (position - 1) > 0 ? (list.get(position - 1)
66 .getName().substring(0, 1).charAt(0) + "") : "";
67 if (!lastLetter.equals(currentLetter)) {
68 holder.vip_friend_letter.setText(currentLetter);
69 holder.vip_friend_letter.setVisibility(View.VISIBLE);
70 } else {
71 holder.vip_friend_letter.setVisibility(View.GONE);
72
73 }
74
75 return convertView;
76 }
77
78 public class viewHolder {
79 TextView vip_friend_letter;
80 ImageView vip_friend_logo;
81 TextView vip_friend_name;
82 }
83
84 }
View Code
3.entity

![]()
1 package com.anna.contact.entity;
2
3 import android.widget.ImageView;
4
5 public class Friend {
6 private String name;
7 private String letter;
8 private ImageView logo;
9
10 public String getName() {
11 return name;
12 }
13
14 public void setName(String name) {
15 this.name = name;
16 }
17
18 public String getLetter() {
19 return letter;
20 }
21
22 public void setLetter(String letter) {
23 this.letter = letter;
24 }
25
26 public ImageView getLogo() {
27 return logo;
28 }
29
30 public void setLogo(ImageView logo) {
31 this.logo = logo;
32 }
33
34 }
View Code
4.view

![]()
1 package com.anna.contact.view;
2
3 import android.content.Context;
4 import android.graphics.Canvas;
5 import android.graphics.Color;
6 import android.graphics.Paint;
7 import android.graphics.Typeface;
8 import android.util.AttributeSet;
9 import android.view.MotionEvent;
10 import android.view.View;
11
12 public class LetterlistViewForFriend extends View {
13
14 public LetterlistViewForFriend(Context context, AttributeSet attrs)
15 {
16 super(context, attrs);
17 // TODO Auto-generated constructor stub
18 }
19 public LetterlistViewForFriend(Context context)
20 {
21 super(context);
22 }
23
24 public LetterlistViewForFriend(Context context, AttributeSet attrs, int defStyle)
25 {
26 super(context, attrs, defStyle);
27 }
28
29 OnTouchingLetterChangedListener onTouchingLetterChangedListener;
30 String[] b =
31 {"A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z" };
32 int choose = -1;
33 Paint paint = new Paint();
34
35 boolean showBkg = false;
36
37 @Override
38 protected void onDraw(Canvas canvas)
39 {
40 super.onDraw(canvas);
41
42 if (showBkg)
43 {
44 canvas.drawColor(Color.parseColor("#40000000"));
45 }
46
47 int height = getHeight();
48 int width = getWidth();
49 int singleHeight = height / b.length;
50 for (int i = 0; i < b.length; i++)
51 {
52 //paint.setTextSize(19) ;
53 paint.setColor(Color.BLACK);
54 paint.setTypeface(Typeface.DEFAULT_BOLD);
55
56 paint.setAntiAlias(true);
57 if (i == choose)
58 {
59 paint.setColor(Color.parseColor("#3399ff"));
60 paint.setFakeBoldText(true);
61 }
62 float xPos = width / 2 - paint.measureText(b[i]) / 2;
63 float yPos = singleHeight * i + singleHeight;
64
65 canvas.drawText(b[i], xPos, yPos, paint);
66 paint.reset();
67 }
68
69 }
70
71 @Override
72 public boolean dispatchTouchEvent(MotionEvent event)
73 {
74 final int action = event.getAction();
75 final float y = event.getY();
76 final int oldChoose = choose;
77 final OnTouchingLetterChangedListener listener = onTouchingLetterChangedListener;
78 final int c = (int) (y / getHeight() * b.length);
79
80 switch (action)
81 {
82 case MotionEvent.ACTION_DOWN:
83 showBkg = true;
84 if (oldChoose != c && listener != null)
85 {
86 if (c > 0 && c < b.length)
87 {
88 listener.onTouchingLetterChanged(b[c]);
89 choose = c;
90 invalidate();
91 }
92 }
93
94 break;
95 case MotionEvent.ACTION_MOVE:
96 if (oldChoose != c && listener != null)
97 {
98 if (c > 0 && c < b.length)
99 {
100 listener.onTouchingLetterChanged(b[c]);
101 choose = c;
102 invalidate();
103 }
104 }
105 break;
106 case MotionEvent.ACTION_UP:
107 showBkg = true;
108 if (oldChoose != c && listener != null)
109 {
110 if (c > 0 && c < b.length)
111 {
112 listener.onTouchingLetterChanged(b[c]);
113 choose = c;
114 invalidate();
115 }
116 }
117
118 break;
119 }
120 return true;
121 }
122
123
124 @Override
125 public boolean onTouchEvent(MotionEvent event)
126 {
127 return super.onTouchEvent(event);
128 }
129
130 public void setOnTouchingLetterChangedListener(OnTouchingLetterChangedListener onTouchingLetterChangedListener)
131 {
132 this.onTouchingLetterChangedListener = onTouchingLetterChangedListener;
133 }
134
135 public interface OnTouchingLetterChangedListener
136 {
137 public void onTouchingLetterChanged(String s);
138 }
139 }
View Code
源碼下載:https://yunpan.cn/cr9yS4D6RWwid訪問密碼 09fa