一 、前言
上次模仿開發者頭條首頁實現了一個版本,給345大神,我的產品經理一看,又被鄙視了一把,說還在用老的技術,於是乎這三天把整個design包研究了一遍,然後把首頁的代碼幾乎重寫了一遍。。。。順便用上了android studio,方便大家導入。。。
效果圖如下:
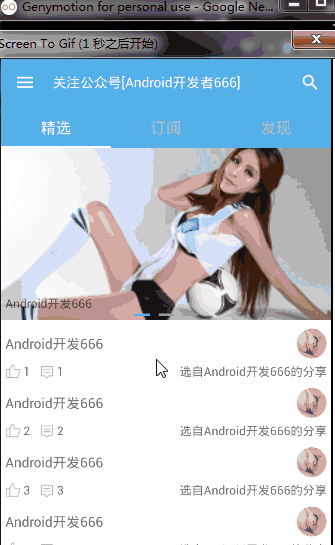
從gif動態效果圖中我們可以看出,跟上次沒有啥變化,唯一變化的就是列表上拉的時候會隱藏標題欄。。。其實裡面的代碼幾乎重寫了一遍,用了Android Design Support Library。
Google在2015的IO大會上,給我們帶來了更加詳細的Material Design設計規范,同時,也給我們帶來了全新的Android Design Support Library,在這個support庫裡面,Google給我們提供了更加規范的MD設計風格的控件。最重要的是,Android Design Support Library的兼容性更廣,直接可以向下兼容到Android 2.2。這不得不說是一個良心之作。
二、Toolbar+TabLayout 實現 標題欄+三個切換Tab
標題欄我之前引用的一個布局文件,現在改成了Toolbar。一個控件就夠了。
三個切換的Tab之前用的三個TextView,現在換成了TabLayout。
換了之後有哪些優點:
1).跟的上時代,逼格提高,更加規范的MD設計風格
2).控件變少了,現在一個功能一個控件就夠
3).點擊Tab文字變色,還有指示器的滑動在xml加個屬性就行。
4).隱藏顯示標題欄很方便。只需要在布局文件中改動就行.
1.布局文件
最外層是CoordinatorLayout,裡面主要就分兩塊,AppBarLayout+ViewPager(AppBarLayout裡面包含標題欄的Toolbar+TabLayout,ViewPager用來切換Fragment顯示)
為了使得Toolbar有滑動效果,必須做到如下三點:
CoordinatorLayout作為布局的父布局容器。
給需要滑動的組件設置 app:layout_scrollFlags=”scroll|enterAlways” 屬性。
滑動的組件必須是AppBarLayout頂部組件。
給滑動的組件設置app:layout_behavior屬性
5.ViewPager顯示的Fragment裡面不能是ListView,必須是RecyclerView。
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
- <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
- xmlns:app="http://schemas.android.com/apk/res-auto"
- android:id="@+id/coordinatorLayout"
- android:layout_width="match_parent"
- android:layout_height="match_parent">
-
- <android.support.design.widget.AppBarLayout
- android:id="@+id/appBarLayout"
- android:layout_width="match_parent"
- android:layout_height="wrap_content">
-
- <android.support.v7.widget.Toolbar
- android:id="@+id/toolbar"
- android:layout_width="match_parent"
- android:layout_height="wrap_content"
- android:background="@color/launcher_item_select"
- app:layout_scrollFlags="scroll|enterAlways"
- app:titleTextAppearance="@style/ansenTextTitleAppearance">
- </android.support.v7.widget.Toolbar>
- <android.support.design.widget.TabLayout
- android:id="@+id/tabLayout"
- android:layout_width="match_parent"
- android:layout_height="wrap_content"
- android:layout_gravity="center_horizontal"
- android:background="@color/main_color"
- app:tabIndicatorColor="@color/white_normal"
- app:tabIndicatorHeight="2dp"
- app:tabSelectedTextColor="@color/main_title_text_select"
- app:tabTextAppearance="@style/AnsenTabLayoutTextAppearance"
- app:tabTextColor="@color/main_title_text_normal"/>
- </android.support.design.widget.AppBarLayout>
-
- <android.support.v4.view.ViewPager
- android:id="@+id/viewPager"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- app:layout_behavior="@string/appbar_scrolling_view_behavior" />
- </android.support.design.widget.CoordinatorLayout>
2.代碼實現 MainFragment.java
1).初始化Toolbar,加載menu布局,實現標題欄的自定義。給NavigationIcon設置點擊事件等。下面貼出代碼實現,還有menu布局文件我就不貼出來了。那個也沒啥技術含量。
Java代碼
- Toolbar toolbar = (Toolbar) rootView.findViewById(R.id.toolbar);
- toolbar.inflateMenu(R.menu.ansen_toolbar_menu);
- toolbar.setNavigationIcon(R.mipmap.ic_menu_white);
- toolbar.setTitle("關注公眾號[Android開發者666]");
- toolbar.setTitleTextColor(getResources().getColor(android.R.color.white));
- toolbar.setNavigationOnClickListener(onClickListener);
NavigationIcon監聽函數,回調到MainActivity去。
Java代碼
- private View.OnClickListener onClickListener=new View.OnClickListener(){
- @Override
- public void onClick(View view) {
- if(drawerListener!=null){
- drawerListener.open();
- }
- }
- };
MainActivity.java
首先寫了一個用來回調的接口
Java代碼
- public interface MainDrawerListener{
- public void open();//打開Drawer
- }
初始化Fragment的時候把MainDrawerListener對象傳遞過去 這樣才能實現回調
Java代碼
- mainFragment=new MainFragment(drawerListener);
Java代碼
- private MainDrawerListener drawerListener=new MainDrawerListener() {
- @Override
- public void open() {
- mDrawerLayout.openDrawer(Gravity.LEFT);
- }
- };
2).給ViewPager設置Fragment適配器,給TabLayout綁定ViewPager,這樣ViewPager滑動的時候或者選擇tab的時候都會切換fragment。
Java代碼
- vPager = (ViewPager) rootView.findViewById(R.id.viewPager);
- vPager.setOffscreenPageLimit(2);//設置緩存頁數
- vPager.setCurrentItem(0);
-
- FragmentAdapter pagerAdapter = new FragmentAdapter(getActivity().getSupportFragmentManager());
- SelectedFragment selectedFragment=new SelectedFragment();
- SubscribeFragment subscribeFragment=new SubscribeFragment();
- FindFragment findFragment=new FindFragment();
-
- pagerAdapter.addFragment(selectedFragment,"精選");
- pagerAdapter.addFragment(subscribeFragment,"訂閱");
- pagerAdapter.addFragment(findFragment,"發現");
-
- vPager.setAdapter(pagerAdapter);
-
- TabLayout tabLayout = (TabLayout) rootView.findViewById(R.id.tabLayout);
- tabLayout.setupWithViewPager(vPager);
三 、分析TabLayout切換源碼
我們調用TabLayout的setupWithViewPager(ViewPager viewPager)方法的時候就是設置切換監聽的時候。
Java代碼
- public void setupWithViewPager(ViewPager viewPager) {
- PagerAdapter adapter = viewPager.getAdapter();
- if(adapter == null) {
- throw new IllegalArgumentException("ViewPager does not have a PagerAdapter set");
- } else {
- this.setTabsFromPagerAdapter(adapter);
- viewPager.addOnPageChangeListener(new TabLayout.TabLayoutOnPageChangeListener(this));
- this.setOnTabSelectedListener(new TabLayout.ViewPagerOnTabSelectedListener(viewPager));
- }
- }
從上面代碼中我們可以看到主要設置了兩個監聽函數。先說第一個。
在TabLayout裡面有一個靜態類TabLayoutOnPageChangeListener,用來處理ViewPager改變狀態(切換或者增加)監聽,看過我第三篇文章的同學對ViewPager的狀態改變監聽應該很熟悉了。
Java代碼
- viewPager.addOnPageChangeListener(new TabLayout.TabLayoutOnPageChangeListener(this));
TabLayoutOnPageChangeListener實現了ViewPagerde 的OnPageChangeListener接口,在onPageSelected方法中調用了當前選中的某個Tab的select方法。
Java代碼
- public void onPageSelected(int position) {
- TabLayout tabLayout = (TabLayout)this.mTabLayoutRef.get();
- if(tabLayout != null) {
- tabLayout.getTabAt(position).select();
- }
-
- }
然後繼續跟蹤TabLayout.Tab類的select() 看看如何實現的。我們可以看到又調用了父類(TabLayout)的selectTab。
Java代碼
- public void select() {
- this.mParent.selectTab(this);
- }
然後跟蹤selectTab方法,這裡大家可以看到參數是某個具體Tab對象,首先判斷是不是當前tab,如果不是設置選擇當前的tab,開啟tab滑動動畫。
Java代碼
- void selectTab(TabLayout.Tab tab) {
- if(this.mSelectedTab == tab) {
- if(this.mSelectedTab != null) {
- if(this.mOnTabSelectedListener != null) {
- this.mOnTabSelectedListener.onTabReselected(this.mSelectedTab);
- }
-
- this.animateToTab(tab.getPosition());
- }
- } else {
- int newPosition = tab != null?tab.getPosition():-1;
- this.setSelectedTabView(newPosition);
- if((this.mSelectedTab == null || this.mSelectedTab.getPosition() == -1) && newPosition != -1) {
- this.setScrollPosition(newPosition, 0.0F, true);
- } else {
- this.animateToTab(newPosition);
- }
-
- if(this.mSelectedTab != null && this.mOnTabSelectedListener != null) {
- this.mOnTabSelectedListener.onTabUnselected(this.mSelectedTab);
- }
-
- this.mSelectedTab = tab;
- if(this.mSelectedTab != null && this.mOnTabSelectedListener != null) {
- this.mOnTabSelectedListener.onTabSelected(this.mSelectedTab);
- }
- }
-
- }
上面的代碼我就不一一解釋了,直接看最下面那兩行代碼。調用tab的選擇方法。
Java代碼
- if(this.mSelectedTab != null && this.mOnTabSelectedListener != null) {
- this.mOnTabSelectedListener.onTabSelected(this.mSelectedTab);
- }
選擇監聽的接口
Java代碼
- public interface OnTabSelectedListener {
- void onTabSelected(TabLayout.Tab var1);
-
- void onTabUnselected(TabLayout.Tab var1);
-
- void onTabReselected(TabLayout.Tab var1);
- }
在TabLayout內部實現了OnTabSelectedListener接口,在onTabSelected方法中調用了ViewPager的setCurrentItem(),這個方法大家應該都熟悉吧,我就不多做解釋了。
Java代碼
- public static class ViewPagerOnTabSelectedListener implements TabLayout.OnTabSelectedListener {
- private final ViewPager mViewPager;
-
- public ViewPagerOnTabSelectedListener(ViewPager viewPager) {
- this.mViewPager = viewPager;
- }
-
- public void onTabSelected(TabLayout.Tab tab) {
- this.mViewPager.setCurrentItem(tab.getPosition());
- }
-
- public void onTabUnselected(TabLayout.Tab tab) {
- }
-
- public void onTabReselected(TabLayout.Tab tab) {
- }
- }
上面說到了第一種監聽,就是ViewPager滑動的時候如何切換item,如果切換tab。現在來說第二種情況,就是點擊選擇tab的時候。如何切換的。繼續回到TabLayout的setupWithViewPager(ViewPager viewPager)方法。
Java代碼
- this.setOnTabSelectedListener(new TabLayout.ViewPagerOnTabSelectedListener(viewPager));
看到ViewPagerOnTabSelectedListener類是不是很熟悉,其實就是第一種方法最後調用的那個類。。。。。因為點擊某個tab的時候,tab切換的代碼已經運行,所以我們這裡只需要設置下ViewPager當前選中的item就行。
從源碼分析的文章第一次寫,不知道這樣寫出來大家看的懂麼,還有不對的地方也歡迎大家提出,可以給我評論哦,我會第一時間回復大家。
四 、劇透
本來打算順便寫下RecyclerView的實現的,但是發現內容已經不少了,那就留著下篇文章吧,下篇文章打算左滑裡面的布局用NavigationView實現。然後加上RecyclerView吧。