前面兩部分分別講了RSS概述和解析XML文件,本節講解怎樣在列表中顯示RSS內容。
首先修改main.java文件,調用前面的類,由intentert獲取rss列表並顯示在UI上:
Java代碼
- public final String RSS_URL = "http://blog.sina.com.cn/rss/1267454277.xml";//從網絡獲取RSS地址
- public final String tag = "RSSReader";
- private RSSFeed feed = null;
-
- /** Called when the activity is first created. */
- public void onCreate(Bundle icicle) {
- super.onCreate(icicle);
- setContentView(R.layout.main);
- feed = getFeed(RSS_URL);//調用getFeed方法,從服務器取得rss提要
- showListView(); //把rss內容綁定到ui界面進行顯示
- }
- private RSSFeed getFeed(String urlString) //該方法通過url獲得xml並解析xml內容為RSSFeed對象
- {
- try
- {
- URL url = new URL(urlString);
- SAXParserFactory factory = SAXParserFactory.newInstance();
- SAXParser parser = factory.newSAXParser();
- XMLReader xmlreader = parser.getXMLReader();
- RSSHandler rssHandler = new RSSHandler();
- xmlreader.setContentHandler(rssHandler);
- InputSource is = new InputSource(url.openStream());
- xmlreader.parse(is);
- return rssHandler.getFeed();
- }
- catch (Exception ee)
- {
- return null;
- }
- }
- private void showListView()
- {
- ListView itemlist = (ListView) findViewById(R.id.itemlist);
- if (feed == null)
- {
- setTitle("訪問的RSS無效");
- return;
- }
-
- SimpleAdapter adapter = new SimpleAdapter(this, feed.getAllItemsForListView(),
- android.R.layout.simple_list_item_2, new String[] { RSSItem.TITLE,RSSItem.PUBDATE },
- new int[] { android.R.id.text1 , android.R.id.text2});
- itemlist.setAdapter(adapter); //listview綁定適配器
- itemlist.setOnItemClickListener(this); //設置itemclick事件代理
- itemlist.setSelection(0);
-
- }
-
- public void onItemClick(AdapterView parent, View v, int position, long id) //itemclick事件代理方法
- {
- Intent itemintent = new Intent(this,ActivityShowDescription.class);//構建一個“意圖”,用於指向activity :detail
- Bundle b = new Bundle(); //構建buddle,並將要傳遞參數都放入buddle
- b.putString("title", feed.getItem(position).getTitle());
- b.putString("description", feed.getItem(position).getDescription());
- b.putString("link", feed.getItem(position).getLink());
- b.putString("pubdate", feed.getItem(position).getPubDate());
- itemintent.putExtra("android.intent.extra.rssItem", b); //用android.intent.extra.INTENT的名字來傳遞參數
- startActivityForResult(itemintent, 0);
- }
-
- }
到此,程序已經可以顯示第1個Activity(頁面)了。但由於程序使用了網絡,我們還必須在AndroidManifest.xml中增加使用網絡的權限。
當我們點擊一個item的時候,頁面要跳轉到另一個Activity,用來展現item裡面的內容,所以需要建立一個新的Activity:ActivityShowDescription。
Java代碼
- public class ActivityShowDescription extends Activity {
- public void onCreate(Bundle icicle) {
- super.onCreate(icicle);
- setContentView(R.layout.showdescription);
- String content = null;
- Intent startingIntent = getIntent();
-
-
- if (startingIntent != null) {
- Bundle bundle = startingIntent
- .getBundleExtra("android.intent.extra.rssItem");
- if (bundle == null) {
- content = "不好意思程序出錯啦";
- } else {
- content = bundle.getString("title") + "\n\n"
- + bundle.getString("pubdate") + "\n\n"
- + bundle.getString("description").replace('\n', ' ')
- + "\n\n詳細信息請訪問以下網址:\n" + bundle.getString("link");
- }
- } else {
- content = "不好意思程序出錯啦";
- }
-
- TextView textView = (TextView) findViewById(R.id.content);
- textView.setText(content);
-
- Button backbutton = (Button) findViewById(R.id.back);
-
- backbutton.setOnClickListener(new Button.OnClickListener() {
- public void onClick(View v) {
- finish();
- }
- });
- }
- }
它對應的xml文件如下:
XML/HTML代碼
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- >
- <TextView
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:autoLink="all"
- android:text=""
- android:id="@+id/content"
- android:layout_weight="1.0"
- />
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:text="返回"
- android:id="@+id/back"
- />
- </LinearLayout>
此外還需要在AndroidMainfest.xml裡添加<activity android:name=".ActivityShowDescription"></activity>
這樣我們就完成了這個RSS客戶端小程序,運行效果如下:
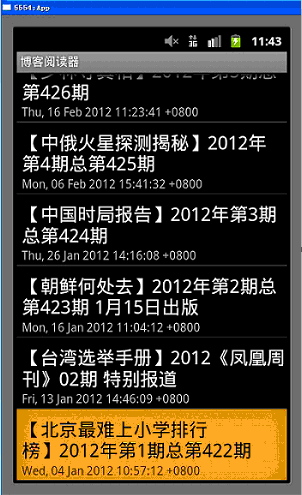
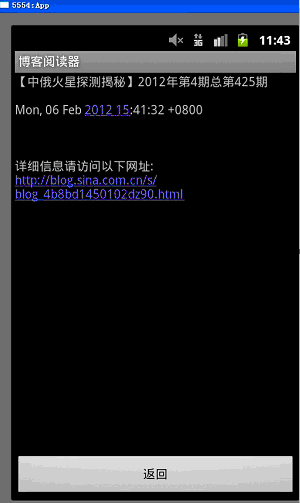
Android系統中RSS客戶端的開發還不算很難吧?以後我們可以做自己的Android RSS閱讀器了。